


The impact of code refactoring on C++ algorithm efficiency and practical suggestions
Code refactoring can affect C++ algorithm efficiency through loop unrolling, function inlining, local variable optimization, and data structure optimization, thereby improving performance and reducing program running time. Practical cases show that the optimized Fibonacci sequence implementation is much faster than the unoptimized version. To optimize performance, it is recommended to identify algorithm bottlenecks, explore refactoring techniques, benchmark improvements, and regularly review and maintain refactored code.
The impact of code refactoring on C++ algorithm efficiency
Code refactoring is a technique to improve code quality, but what impact does it have on algorithm efficiency? ? This article explores the impact of code refactoring on C++ algorithm efficiency and provides practical examples to support our findings.
Factors affecting efficiency
Code refactoring can affect efficiency in the following ways:
- Loop unrolling: Unrolling the loop can reduce branches jump, thus increasing the speed of the algorithm.
- Function inlining: Inlining functions can eliminate function call overhead, thereby reducing program running time.
- Local variable optimization: By promoting local variables to the function scope, parameter passing overhead can be reduced and performance improved.
- Data structure optimization: Optimizing the data structure can reduce the complexity of the algorithm and thereby improve efficiency.
Practical Case
In order to demonstrate the impact of code refactoring on algorithm efficiency, we benchmarked the following two Fibonacci sequences implemented in C++:
// 未优化版本 int fibonacci(int n) { if (n <= 1) { return 1; } else { return fibonacci(n - 1) + fibonacci(n - 2); } } // 优化版本 int fibonacci_optimized(int n) { int f[n + 1]; f[0] = 0; f[1] = 1; for (int i = 2; i <= n; i++) { f[i] = f[i - 1] + f[i - 2]; } return f[n]; }
The following are the benchmark results:
Input size | Unoptimized version time (ms) | Optimized version time (ms) ) |
---|---|---|
10 | 0.0003 | 0.0001 |
20 | 0.0029 | 0.0002 |
30 | 0.0257 | 0.0003 |
40 | 0.2212 | 0.0005 |
1.9008 | 0.0006 |
- Identify the performance bottlenecks of your algorithm.
- Explore refactoring techniques such as loop unrolling, function inlining, and data structure optimization.
- Implement refactoring and benchmark performance improvements.
- After optimization, the refactored code is continuously reviewed and maintained to ensure long-term efficiency.
The above is the detailed content of The impact of code refactoring on C++ algorithm efficiency and practical suggestions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










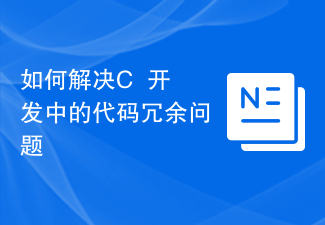
How to solve the code redundancy problem in C++ development. Code redundancy means that when writing a program, there are similar or repeated codes in multiple places. This problem not only makes the code difficult to maintain and read, but also increases the size and complexity of the code. For C++ developers, it is particularly important to solve the problem of code redundancy, because C++ is a powerful programming language, but it can also easily lead to code duplication. The root cause of code redundancy problems lies in unreasonable design and coding habits. To solve this problem, you can start from the following aspects: Use functions and classes: C
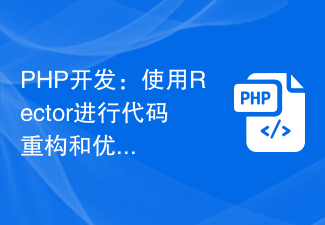
As time passes and requirements change, a project's code can easily become obsolete and difficult to maintain and extend. In PHP development, refactoring is considered one of the necessary tasks to improve code quality and development efficiency. In this process, using the Rector tool can greatly simplify code reconstruction and optimization work. Rector is an open source PHP code reconstruction tool that can help PHP developers automate code reconstruction and optimization, allowing developers to focus more on business development and function implementation. pass
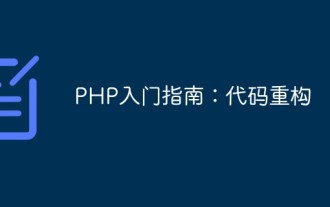
Refactoring is a very important process when writing PHP code. As an application grows, the code base becomes larger and harder to read and maintain. Refactoring is to solve this problem and make the code more modular and better organized and extensible. When we refactor the code, we need to consider the following aspects: Code style Code style is a very important point. Keeping your coding style consistent will make your code easier to read and maintain. Please follow PHP coding standards and be consistent. Try using a code style checking tool such as PHP
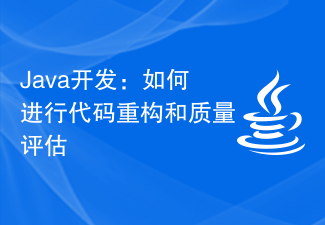
Java Development: Code Refactoring and Quality Assessment Introduction: In the process of software development, code refactoring is one of the important means to improve code quality and maintainability. By refactoring the code, the code can be made more elegant, concise, and easy to understand and modify. However, refactoring is not just about simply modifying the code, but a process that requires rational and systematic thinking. This article will introduce how to perform code refactoring and illustrate it with specific code examples. We will also discuss how to evaluate code quality and why evaluation is important. Heavy code
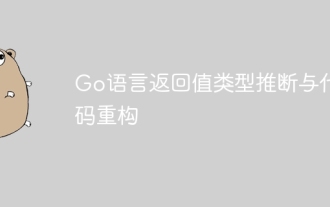
Go language return value type inference automatically infers function return value types, simplifying code and improving readability. The return value type can be omitted and the compiler will automatically infer the type based on the actual return value in the function body. Can be used to refactor existing code to eliminate explicit type declarations. For example, the function calculateTotal that calculates the sum of an array of integers can be refactored into: funccalculateTotal(items[]int){}.
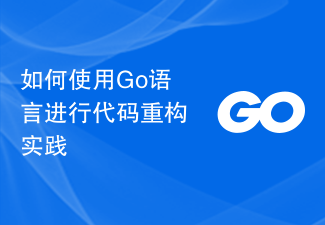
How to use Go language for code refactoring practice introduction: In the software development process, we often face the challenge of code refactoring. Code refactoring refers to optimizing and restructuring existing code to improve code quality and maintainability. This article will introduce how to use Go language for code refactoring practice, and come with corresponding code examples. 1. Principles of code refactoring Before code refactoring, we need to clarify some principles to ensure the smooth progress of refactoring. The following are some important code refactoring principles: Maintain the consistency of code functionality: After refactoring
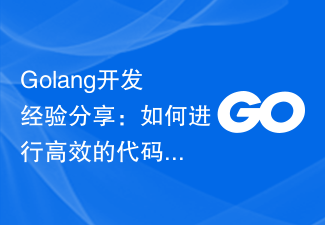
Golang development experience sharing: How to perform efficient code refactoring As the software development process advances, code refactoring becomes increasingly important. Whether it is to improve the readability of the code, enhance the maintainability of the code, or to respond to changes in business requirements, code refactoring is required. In Golang development, how to carry out efficient code refactoring is a question worth exploring. This article will share some experience and techniques in Golang code refactoring, hoping to help readers improve the quality and efficiency of code in actual development. one,
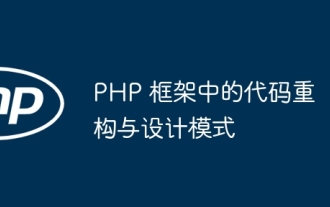
Code refactoring is a process of optimizing software structure, involving techniques such as renaming and extraction methods. Design patterns are general-purpose solutions to common software problems, such as the Singleton pattern and the Observer pattern. By refactoring and using design patterns, you can improve the maintainability, readability, and scalability of your code.
