How to quickly develop web applications using the golang framework
Use the Gin framework to quickly develop Web applications in Go: Install Gin: go get github.com/gin-gonic/gin Create a Web server: Create a Gin router Add a route Run the server Practical case: Create a RESTful API: Add a GET route Get the list of people Add a POST route Create a new person
How to use the Gin framework to quickly develop web applications in Go
Gin is a popular and lightweight Go web framework known for its simple API and high performance. Here's how to use Gin to quickly develop web applications:
Install Gin
go get github.com/gin-gonic/gin
Create a web server
package main import ( "github.com/gin-gonic/gin" ) func main() { // 创建 Gin 路由器 router := gin.Default() // 添加路由 router.GET("/", func(c *gin.Context) { c.String(200, "Hello, World!") }) // 运行服务器 router.Run(":8080") }
Practical Case: Creating a RESTful API
Here's how to use Gin to create a route for a simple RESTful API:
package main import ( "github.com/gin-gonic/gin" "github.com/google/uuid" ) type Person struct { ID uuid.UUID `json:"id"` Name string `json:"name"` } func main() { router := gin.Default() // 添加 GET 路由 router.GET("/people", func(c *gin.Context) { // 获取所有人的列表 people := []Person{} c.JSON(200, people) }) // 添加 POST 路由 router.POST("/people", func(c *gin.Context) { var newPerson Person if err := c.BindJSON(&newPerson); err != nil { c.JSON(400, gin.H{"error": err.Error()}) return } newPerson.ID = uuid.New() // 保存新的人 c.JSON(201, newPerson) }) // 运行服务器 router.Run(":8080") }
Conclusion (remove from the prompt)
It is very easy to quickly develop web applications in Go using the Gin framework. The intuitive API and high performance it offers make it a popular choice, especially for applications that require high throughput.
The above is the detailed content of How to quickly develop web applications using the golang framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










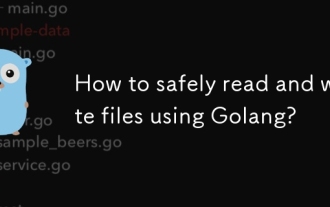
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
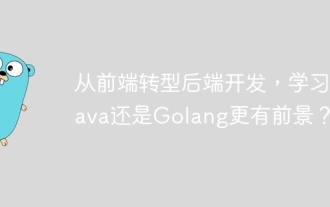
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...

HTML, CSS and JavaScript are the three pillars of web development. 1. HTML defines the web page structure and uses tags such as, etc. 2. CSS controls the web page style, using selectors and attributes such as color, font-size, etc. 3. JavaScript realizes dynamic effects and interaction, through event monitoring and DOM operations.

PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
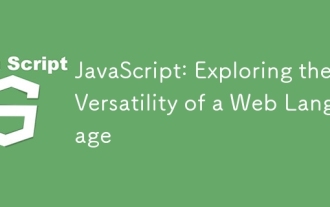
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
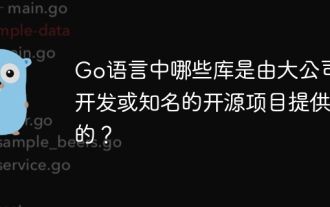
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
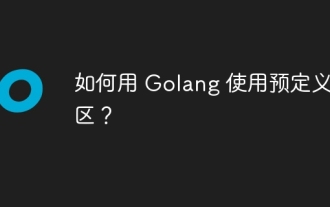
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.

The future trends of HTML are semantics and web components, the future trends of CSS are CSS-in-JS and CSSHoudini, and the future trends of JavaScript are WebAssembly and Serverless. 1. HTML semantics improve accessibility and SEO effects, and Web components improve development efficiency, but attention should be paid to browser compatibility. 2. CSS-in-JS enhances style management flexibility but may increase file size. CSSHoudini allows direct operation of CSS rendering. 3.WebAssembly optimizes browser application performance but has a steep learning curve, and Serverless simplifies development but requires optimization of cold start problems.
