


How to implement function template reuse in generic programming in C++?
Generic programming in C++ is implemented through function templates, making the code independent of data types and reusable. Function templates are general-purpose functions whose arguments are specified as type names and can handle any type of data. By using function template reuse, you can achieve code reusability, reduce redundancy, improve scalability, and create efficient and flexible C++ code.
Generic programming in C++: Implementing function template reuse
Generic programming is a technique for writing code. Allowing it to work on multiple data types independently of the concrete type. In C++, generic programming can be implemented through function templates.
Function template
A function template is a general function that can handle any type of data. To create a function template, use the following syntax:
template<typename T> T add(T a, T b) { return a + b; }
typename T
Specifies that the argument to the template is a type name.
Practical Case
Suppose we have a function that adds two numbers. Using generic programming, we can write a general function that can handle any type of number:
#includetemplate<typename T> T add(T a, T b) { return a + b; } int main() { int x = 5; int y = 3; std::cout << add(x, y) << '\n'; // 输出 8 double d1 = 3.14; double d2 = 2.71; std::cout << add(d1, d2) << '\n'; // 输出 5.85 }
In this example, the add() function accepts two types of template parameters T and can be used to combine the two types. Add numbers of different types.
Advantages
Function template reuse provides many advantages, including:
- Code reusability: You can reuse common functions on multiple data types.
- Reduce code redundancy: You don’t need to write a separate function for each data type.
- Extensibility: When adding new data types, you don’t have to modify existing code.
By using function templates, you can create efficient, flexible, and reusable C++ code.
The above is the detailed content of How to implement function template reuse in generic programming in C++?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics











1. What is generic programming? Generic programming refers to the implementation of a common data type in a programming language so that this data type can be applied to different data types, thereby achieving code reuse and efficiency. PHP is a dynamically typed language. It does not have a strong type mechanism like C++, Java and other languages, so it is not easy to implement generic programming in PHP. 2. Generic programming in PHP There are two ways to implement generic programming in PHP: using interfaces and using traits. Create an interface in PHP using an interface
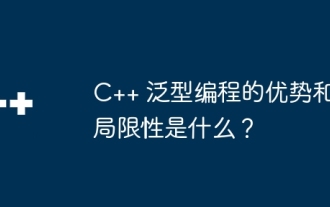
Generic programming is a C++ technology that has the following advantages: improves code reusability and can handle multiple data types. The code is more concise and easier to read. Improves efficiency in some cases. But it also has limitations: it takes more time to compile. The compiled code will be larger. There may be runtime overhead.
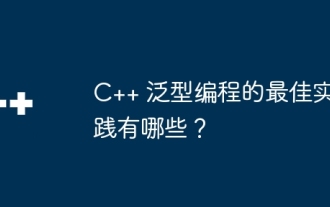
Best practices for C++ generic programming include explicitly specifying type requirements for type parameters. Avoid using empty type parameters. Follow the Liskov substitution principle to ensure that the subtype has the same interface as the parent type. Limit the number of template parameters. Use specializations with caution. Use generic algorithms and containers. Use namespaces to organize code.
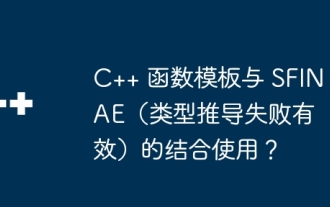
Function templates are used in conjunction with SFINAE to create generic functions and adjust function behavior based on template parameter types. SFINAE allows us to control function availability based on whether template parameter type deduction fails. When used together, function templates can refine behavior based on type constraints, such as distinguishing between integer and non-integer types, excluding boolean types, etc., resulting in flexible and type-safe code.

Function templates improve code reusability by defining generic functions, thereby optimizing C++ code: Function template basics: Defining functions using generic type parameters allows the function to be applied to different data types. Practical case: The findMax function template can be used for any array type to find the maximum value, avoiding repeated code of writing functions of different data types. Performance optimization: Instantiating function templates of specific types supports compiler optimizations, such as inlining and eliminating virtual function calls, reducing function call overhead.
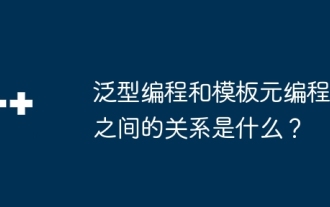
Generic programming and template metaprogramming are two powerful techniques in modern C++ for processing different types of data at runtime (generic programming) and creating and evaluating code at compile time (template metaprogramming). Although they are both based on templates, they are very different in functionality and usage. In practice, the two techniques are often used together, for example, generic code can be combined with template metaprogramming to create and instantiate data structures at runtime.
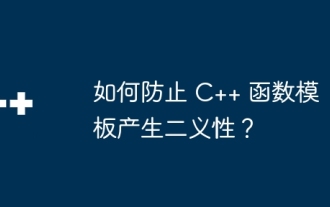
To prevent ambiguity in C++ function templates, solutions include explicitly specifying template parameters, by specifying a type parameter list in the function call. Use auxiliary templates to simplify the call when the function template has many parameters. This is achieved by creating an auxiliary template that accepts different types of parameters and using this template to simplify the call.
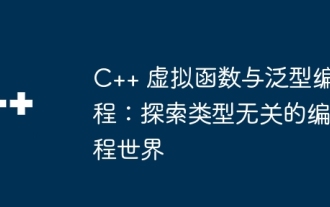
Virtual functions and generic programming are features in C++ for creating type-independent and extensible code. Virtual functions allow derived classes to override methods in a base class, thereby achieving polymorphic behavior. Generic programming involves creating algorithms and data structures that are not bound to a specific type, using type parameters to represent abstract types. By using virtual functions for polymorphism and generic programming for type-independent operations, developers can build flexible and maintainable software.
