


How to optimize the use of arrays and collections in Java to improve performance?
Optimize Java array and collection performance: For different types of elements, use basic type arrays (same type) or object arrays (different types). According to the application requirements, choose the appropriate collection class: List stores ordered elements, Set stores non-repeating elements, and Map stores key-value pairs. Optimize collection traversal: Using Iterator is more efficient than for-each loop and avoids the creation of intermediate collections. Use best practices: pre-allocate collections, use streaming APIs, avoid immutable collections, leverage parallel streams.
Optimizing the use of arrays and collections in Java to improve performance
Preface
In Java, optimizing the use of arrays and collections is critical to improving application performance. By choosing the right data structures, leveraging the Java Collections Framework, and adopting best practices, you can significantly improve the efficiency of your code.
Use an appropriate array
- Basic type array: For scenarios where a large number of elements of the same type need to be stored (such as int[]) , please use basic type array. It is faster and takes up less space than an array of objects.
- Object array: If you need to store elements of different types, use Object[]. However, it is slower than an array of primitive types and takes up more space.
Choose the appropriate collection
The Java Collections Framework provides a variety of collection classes, each with its own unique characteristics:
- List: Used to store a collection of ordered elements, such as ArrayList.
- Set: A collection used to store non-repeating elements, such as HashSet.
- Map: Used to store a collection of key-value pairs, such as HashMap.
Depending on the specific needs of your application, choosing the most appropriate collection can significantly improve performance.
Practical case: Optimizing collection traversal
The following example shows how to improve performance by optimizing collection traversal:
List<Integer> list = new ArrayList<>(); // 使用普通 for-each 循环遍历 for (int num : list) { // 处理元素 }
This can be optimized as:
List<Integer> list = new ArrayList<>(); // 使用 Iterator 遍历 Iterator<Integer> iterator = list.iterator(); while (iterator.hasNext()) { // 处理元素 }
Iterator traversal is faster than for-each loop because it does not create intermediate collections.
Best Practices
- Use pre-allocated collections to avoid frequent reallocation operations.
- Use the streaming API introduced in Java 8 and higher for efficient data processing.
- Avoid using immutable collections (such as Collections.unmodifiableList()) as it causes additional overhead.
- When possible, use parallel streams to take full advantage of multi-core processors.
The above is the detailed content of How to optimize the use of arrays and collections in Java to improve performance?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










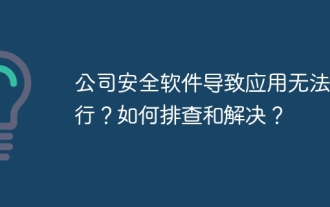
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
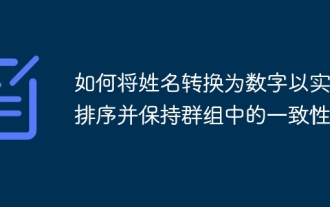
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
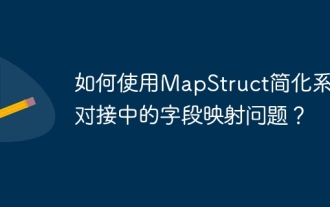
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
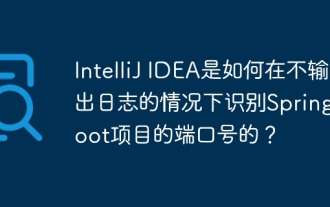
Start Spring using IntelliJIDEAUltimate version...
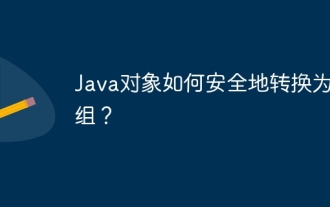
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
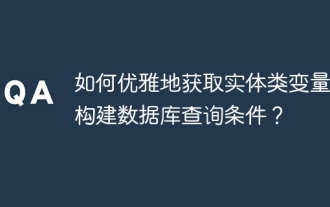
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
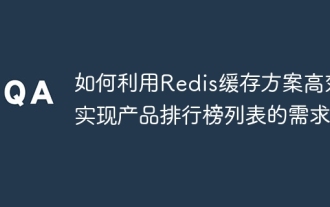
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
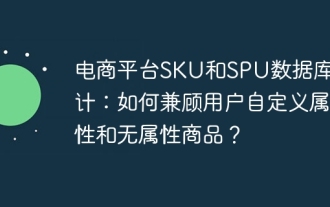
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
