【MongoDB数据库】JavaMongoDBCRUDExample
上一篇我们讲了MongoDB 的命令入门初探,本篇blog将基于上一篇blog所建立的数据库和表完成一个简单的Java MongoDB CRUD Example,利用Java连接MongoDB数据库,并实现创建数据库、获取表、遍历表中的对象、对表中对象进行CRUD操作等例程。 1、下载MongoDB Jav
上一篇我们讲了MongoDB 的命令入门初探,本篇blog将基于上一篇blog所建立的数据库和表完成一个简单的Java MongoDB CRUD Example,利用Java连接MongoDB数据库,并实现创建数据库、获取表、遍历表中的对象、对表中对象进行CRUD操作等例程。
1、下载MongoDB Java 支持驱动包
【gitHub下载地址】https://github.com/mongodb/mongo-java-driver/downloads
2、建立Java工程,并导入jar包
3、连接本地数据库服务器
在控制面板中开启Mongodb服务,具体操作可参考【MongoDB数据库】如何安装、配置MongoDB
try { mongo = new MongoClient("localhost", 27017);// 保证MongoDB服务已经启动 db = mongo.getDB("andyDB");// 获取到数据库 } catch (UnknownHostException e) { e.printStackTrace(); }
3、遍历所有的数据库名
public class DBConnection extends TestCase { private MongoClient mongo; private DB db ; @Override protected void setUp() throws Exception { // TODO Auto-generated method stub super.setUp(); try { mongo = new MongoClient("localhost", 27017);// 保证MongoDB服务已经启动 db = mongo.getDB("andyDB");// 获取到数据库andyDB } catch (UnknownHostException e) { e.printStackTrace(); } } public void testGetAllDB() { List<String> dbs = mongo.getDatabaseNames();// 获取到所有的数据库名 for (String dbname : dbs) { System.out.println(dbname); } } }
4、获取到指定数据库
DB db = mongo.getDB("andyDB");// 获取到数据库
5、遍历数据库中所有的表名
在DBConnection测试类中添加如下测试方法即可:
public void testGetAllTables() { Set<String> tables = db.getCollectionNames(); for (String coll : tables) { System.out.println(coll); } }
6、获取到指定的表
DBCollection table = db.getCollection("person");
7、遍历表中所有的对象
public void testFindAll(){ DBCollection table = db.getCollection("person"); DBCursor dbCursor = table.find(); while(dbCursor.hasNext()){ DBObject dbObject = dbCursor.next(); //打印该对象的特定字段信息 System.out.println("name:"+ dbObject.get("name")+",age:"+dbObject.get("age")); //打印该对象的所有信息 System.out.println(dbObject); } }
Console窗口打印消息:
name:jack,age:50.0
{ "_id" : { "$oid" : "537761da2c82bf816b34e6cf"} , "name" : "jack" , "age" : 50.0}
name:小王,age:24
{ "_id" : { "$oid" : "53777096d67d552056ab8916"} , "name" : "小王" , "age" : 24}
8、保存对象
1)保存对象方法一
public void testSave() { DBCollection table = db.getCollection("person"); BasicDBObject document = new BasicDBObject(); document.put("name", "小郭");// 能直接插入汉字 document.put("age", 24);//"age"对应的值是int型 table.insert(document); }
> db.person.find()
{ "_id" : ObjectId("537761da2c82bf816b34e6cf"), "name" : "jack", "age" : 50 }
{ "_id" : ObjectId("53777096d67d552056ab8916"), "name" : "小王", "age" : 24 }
{ "_id" : ObjectId("5377712cd67d84f62c65c4f6"), "name" : "小郭", "age" : 24 }
2)保存对象方法二
public void testSave2() { DBCollection table = db.getCollection("person"); BasicDBObject document = new BasicDBObject();//可以添加多个字段 document.put("name", "小张");// 能直接插入汉字 document.put("password", "xiaozhang");// 多添加一个字段也是可以的,因为MongoDB保存的是对象; document.put("age", "23");//"age"对应的值是String table.insert(document); }
在mongodb shell中使用命令查看数据
> db.person.find()
{ "_id" : ObjectId("537761da2c82bf816b34e6cf"), "name" : "jack", "age" : 50 }
{ "_id" : ObjectId("53777096d67d552056ab8916"), "name" : "小王", "age" : 24 }
{ "_id" : ObjectId("5377712cd67d84f62c65c4f6"), "name" : "小郭", "age" : 24 }
{ "_id" : ObjectId("53777230d67dfe576de5079a"), "name" : "小张", "password" : "xiaozhang", "age" : "23" }
3)保存对象方法三(通过添加Map集合的方式添加数据到BasicDBObject)
public void testSave3(){ DBCollection table = db.getCollection("person"); Map<String,Object> maps = new HashMap<String,Object>(); maps.put("name", "小李"); maps.put("password", "xiaozhang"); maps.put("age", 24); BasicDBObject document = new BasicDBObject(maps);//这样添加后,对象里的字段是无序的。 table.insert(document); }
在mongodb shell中使用命令查看数据
> db.person.find()
{ "_id" : ObjectId("537761da2c82bf816b34e6cf"), "name" : "jack", "age" : 50 }
{ "_id" : ObjectId("53777096d67d552056ab8916"), "name" : "小王", "age" : 24 }
{ "_id" : ObjectId("5377712cd67d84f62c65c4f6"), "name" : "小郭", "age" : 24 }
{ "_id" : ObjectId("53777230d67dfe576de5079a"), "name" : "小张", "password" : "xiaozhang", "age" : "23" }
{ "_id" : ObjectId("537772e9d67df098a26d79a6"), "age" : 24, "name" : "小李", "password" : "xiaozhang" }
9、更新对象
我们可以结合【mongodb shell命令】db.person.update({name:"小李"},{$set:{password:"hello"}})来理解Java是如何操作对象来更新的。{name:"小李"}是一个BasicDBObject,{$set:{password:"hello"}也是一个BasicDBObject,这样理解的话,你就会觉得mongodb shell命令操作和Java操作很相似。
public void testUpdate() { DBCollection table = db.getCollection("person"); BasicDBObject query = new BasicDBObject(); query.put("name", "小张"); BasicDBObject newDocument = new BasicDBObject(); newDocument.put("age", 23); BasicDBObject updateObj = new BasicDBObject(); updateObj.put("$set", newDocument); table.update(query, updateObj); } // 命令操作:db.person.update({name:"小李"},{$set:{password:"hello"}}) public void testUpdate2() { DBCollection table = db.getCollection("person"); BasicDBObject query = new BasicDBObject("name", "小张"); BasicDBObject newDocument = new BasicDBObject("age", 24); BasicDBObject updateObj = new BasicDBObject("$set", newDocument); table.update(query, updateObj); }
10、删除对象
可参考db.users.remove({name:"小李"})命令来理解Java操作对象
public void testDelete(){ DBCollection table = db.getCollection("person"); BasicDBObject query = new BasicDBObject("name", "小李"); table.remove(query); }
11、参考
Java + MongoDB Hello World Example(推荐)
12、你可能感兴趣
【MongoDB数据库】如何安装、配置MongoDB
【MongoDB数据库】MongoDB 命令入门初探

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










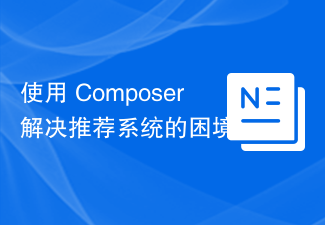
When developing an e-commerce website, I encountered a difficult problem: how to provide users with personalized product recommendations. Initially, I tried some simple recommendation algorithms, but the results were not ideal, and user satisfaction was also affected. In order to improve the accuracy and efficiency of the recommendation system, I decided to adopt a more professional solution. Finally, I installed andres-montanez/recommendations-bundle through Composer, which not only solved my problem, but also greatly improved the performance of the recommendation system. You can learn composer through the following address:

Oracle is not only a database company, but also a leader in cloud computing and ERP systems. 1. Oracle provides comprehensive solutions from database to cloud services and ERP systems. 2. OracleCloud challenges AWS and Azure, providing IaaS, PaaS and SaaS services. 3. Oracle's ERP systems such as E-BusinessSuite and FusionApplications help enterprises optimize operations.
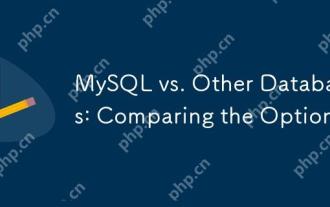
MySQL is suitable for web applications and content management systems and is popular for its open source, high performance and ease of use. 1) Compared with PostgreSQL, MySQL performs better in simple queries and high concurrent read operations. 2) Compared with Oracle, MySQL is more popular among small and medium-sized enterprises because of its open source and low cost. 3) Compared with Microsoft SQL Server, MySQL is more suitable for cross-platform applications. 4) Unlike MongoDB, MySQL is more suitable for structured data and transaction processing.
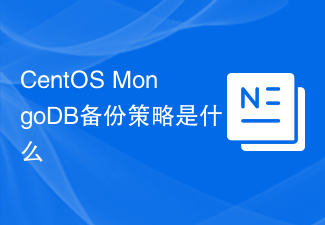
Detailed explanation of MongoDB efficient backup strategy under CentOS system This article will introduce in detail the various strategies for implementing MongoDB backup on CentOS system to ensure data security and business continuity. We will cover manual backups, timed backups, automated script backups, and backup methods in Docker container environments, and provide best practices for backup file management. Manual backup: Use the mongodump command to perform manual full backup, for example: mongodump-hlocalhost:27017-u username-p password-d database name-o/backup directory This command will export the data and metadata of the specified database to the specified backup directory.
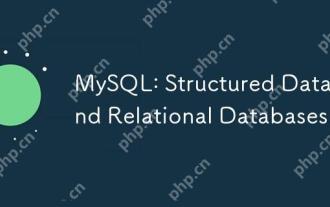
MySQL efficiently manages structured data through table structure and SQL query, and implements inter-table relationships through foreign keys. 1. Define the data format and type when creating a table. 2. Use foreign keys to establish relationships between tables. 3. Improve performance through indexing and query optimization. 4. Regularly backup and monitor databases to ensure data security and performance optimization.
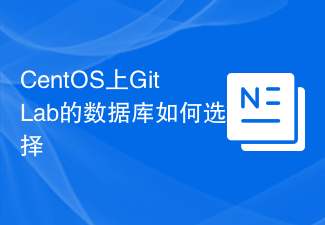
GitLab Database Deployment Guide on CentOS System Selecting the right database is a key step in successfully deploying GitLab. GitLab is compatible with a variety of databases, including MySQL, PostgreSQL, and MongoDB. This article will explain in detail how to select and configure these databases. Database selection recommendation MySQL: a widely used relational database management system (RDBMS), with stable performance and suitable for most GitLab deployment scenarios. PostgreSQL: Powerful open source RDBMS, supports complex queries and advanced features, suitable for handling large data sets. MongoDB: Popular NoSQL database, good at handling sea
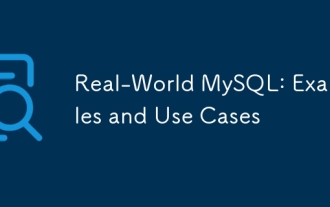
MySQL's real-world applications include basic database design and complex query optimization. 1) Basic usage: used to store and manage user data, such as inserting, querying, updating and deleting user information. 2) Advanced usage: Handle complex business logic, such as order and inventory management of e-commerce platforms. 3) Performance optimization: Improve performance by rationally using indexes, partition tables and query caches.
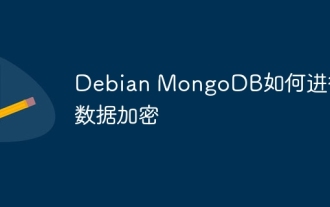
Encrypting MongoDB database on a Debian system requires following the following steps: Step 1: Install MongoDB First, make sure your Debian system has MongoDB installed. If not, please refer to the official MongoDB document for installation: https://docs.mongodb.com/manual/tutorial/install-mongodb-on-debian/Step 2: Generate the encryption key file Create a file containing the encryption key and set the correct permissions: ddif=/dev/urandomof=/etc/mongodb-keyfilebs=512
