C# Learning Diary 22---Multiple Inheritance
Inheritance is one of the most important concepts in object-oriented programming. Inheritance allows us to define a class based on one class to define another class. When a class is derived from another class, the derived class inherits the characteristics from the base class
The idea of inheritance is realized Belongs to (IS-A ) relation. For example, mammals are (IS-A) animals, dogs are (IS-A) mammals, so dogs are (IS-A) animals.
Base class and derived class:
In C#, a derived class inherits members, methods, properties, fields, events, index indicators from its direct base class but except for constructors and destructors function.
Write an example below.
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Test { class Anlimal //定义一个基类 { protected int foot = 4; protected double weight = 22.4; protected void say(string type, string call) { Console.WriteLine("类别:{0},叫声:{1} ",type,call); } } //Dog 继承Anlimal class Dog:Anlimal { static void Main(string[] args) { Dog dog = new Dog(); int foot = dog.foot; double weight = dog.weight; Console.WriteLine("dog foot: {0}\ndog weight:{1}",foot,weight); dog.say("狗", "汪汪"); } } }
Result
Multiple inheritance:
C# does not support multiple inheritance. However, you can use interfaces to implement multiple inheritance. In the above example, we added a smallanlimal interface for it
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Test { class Anlimal //定义一个基类 { protected int foot = 4; protected double weight = 22.4; protected void say(string type, string call) { Console.WriteLine("类别:{0},叫声:{1} ",type,call); } } public interface smallanlimal //添加一个接口 接口只声明方法在子类中实现 { protected void hight(double hight); } //Dog 继承Anlimal class Dog:Anlimal,smallanlimal { public void hight(double hight) //实现接口 { Console.WriteLine("Hight: {0}",hight); } static void Main(string[] args) { Dog dog = new Dog(); int foot = dog.foot; double weight = dog.weight; dog.hight(23.23); Console.WriteLine("dog foot: {0}\ndog weight:{1}",foot,weight); dog.say("狗", "汪汪"); } } }
The above is the content of C# Learning Diary 22---Multiple Inheritance. For more related content, please pay attention to PHP Chinese Net (www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










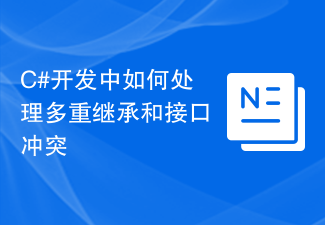
How to deal with multiple inheritance and interface conflicts in C# development requires specific code examples. Although multiple inheritance is not supported in C#, similar functions can be achieved through interfaces. However, using multiple interfaces may lead to conflicting interface methods. In this article, we'll discuss how to handle this situation and provide some practical code examples. Reasons for interface conflicts In C#, a class can implement multiple interfaces. If there are methods with the same name in multiple interfaces, method conflicts will occur. For example, we define two interfaces IInterface1

Python is an object-oriented programming language that supports multiple inheritance. In the process of multiple inheritance, various errors are often encountered, such as the "diamond inheritance" problem, that is, multiple subclasses inherit from the same parent class at the same time. This This will lead to problems such as increased code complexity and difficulty in maintenance. This article will introduce how to solve multiple inheritance errors in Python. 1. Use super() In Python, you can use the super() function to avoid problems caused by multiple inheritance. When calling a parent class method in a subclass, you can
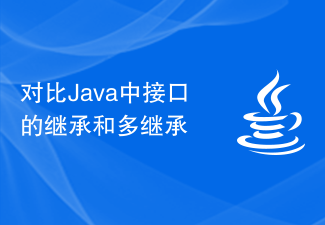
Comparison of Interface Inheritance and Multiple Inheritance in Java In Java, an interface is an abstract type that defines methods and constants. Interfaces can be implemented by classes, and a class can implement multiple interfaces. In the implementation of interfaces, there are two methods: interface inheritance and multiple inheritance. This article will discuss the differences between the two methods and give specific code examples to deepen understanding. Inheritance of interfaces Inheritance of interfaces means that one interface can inherit from another interface, and the methods and constants in the inherited interface will also be inherited. Interface inheritance uses the keyword exte
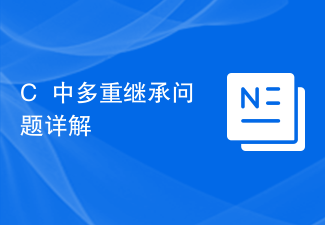
Detailed explanation of multiple inheritance issues in C++ Introduction In C++, multiple inheritance is a feature that allows a derived class to inherit properties and behaviors from multiple base classes. However, since multiple inheritance introduces some complexity, developers must handle it carefully to avoid potential problems. This article will discuss the issue of multiple inheritance in C++ in detail and provide specific code examples. Basic Concepts Multiple inheritance allows a derived class to inherit properties and methods from multiple base classes. For example, we can define a base class called Animal and then define a base class called B
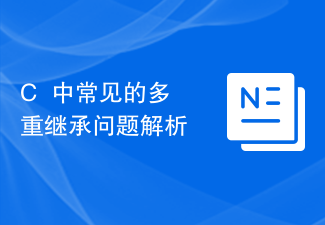
Analysis of Common Multiple Inheritance Problems in C++ Multiple inheritance is a common object-oriented programming technique that allows one class to inherit multiple base classes. However, multiple inheritance often raises issues and challenges that need to be carefully understood and dealt with by developers. Diamond inheritance problem Diamond inheritance means that a derived class inherits two base classes at the same time, and the two base classes jointly inherit the same base class. This inheritance relationship forms a diamond-shaped structure, resulting in the existence of two members in the derived class that are directly or indirectly inherited from the base class. The sample code is as follows: class
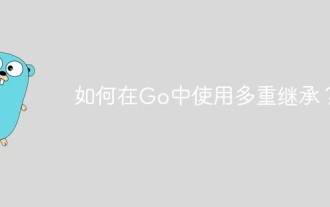
In object-oriented programming, inheritance is an important concept that allows us to reuse existing code more easily. However, in some cases, we need a class to inherit from multiple parent classes at the same time. This is the concept of multiple inheritance. Although multiple inheritance is useful, it is not easy to implement in some programming languages. For example, Java and C# both prohibit multiple inheritance. In contrast, the Go language provides an easy way to use multiple inheritance. This article will introduce how to use multiple inheritance in Go. Go language and multiple inheritance in Go
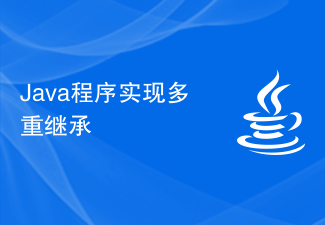
In this article, we will learn how to implement multiple inheritance. Java does not support multiple inheritance. This means that a class cannot extend more than one class, but we can still use the keyword "extends" to achieve the result. AlgorithmStep1–STARTStep2–DeclarethreeclassesnamelyServer,connectionandmy_testStep3–Relatetheclasseswitheachotherusing’extends’keywordStep
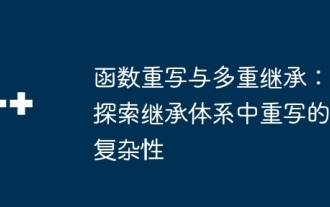
Function overriding and multiple inheritance create complications when used together because it results in child classes inheriting overridden functions from multiple parent classes. The key steps in resolving this problem are as follows: Identify ambiguous overridden methods in subclasses. Use the super() method to explicitly call the implementation of a specific parent class. Call the method of the parent class through super(ParentClass, self).method_name(), where ParentClass is the name of the parent class and self is an instance of the subclass.
