


Use thinkphp5.0 to obtain information, variables, and binding parameters
1. Constructor:
The controller class must inherit the thinkController class to use:
Method_initialize
Code:
<?php namespace appliancontroller;use thinkController;use thinkDb;use thinkRequest;class Index extends Controller { public function _initialize() { echo 'init|||'; } public function hello() { return 'hello'; } }
Look at the output:
2. Prefix method:
['except' => 'Method name, method name']:
means that these methods are not Use the prefix method,
['only' => 'Method name, method name']:
means that only these methods use the prefix method.
*********************************Dividing line********* ***************************
beforeActionList attribute can specify a method as a pre-operation for other methods;
That is, execute before execution;
Code:
<?php namespace appliancontroller;use thinkController;use thinkDb;use thinkRequest;class Index extends Controller {protected $beforeActionList = [ 'first', 'second' => ['except'=>'hello'], 'three' => ['only'=>'hello'], ]; protected function first() { echo 'first<br/>'; } protected function second() { echo 'second<br/>'; } protected function three() { echo 'three<br/>'; } public function hello() { return 'hello'; } }
Look at the output:
It should only output hello, However, because of the pre-operation, three methods are output;
Note: For this operation, the method name must be lowercase;
3. Get URL information
<?php namespace appliancontroller; use thinkController; use thinkDb; use thinkRequest; class Index extends Controller { public function index(){ $request = Request::instance(); // 获取当前域名 echo 'domain: ' . $request->domain() . '<br/>'; // 获取当前入口文件 echo 'file: ' . $request->baseFile() . '<br/>'; // 获取当前URL地址 不含域名 echo 'url: ' . $request->url() . '<br/>'; // 获取包含域名的完整URL地址 echo 'url with domain: ' . $request->url(true) . '<br/>'; // 获取当前URL地址 不含QUERY_STRING echo 'url without query: ' . $request->baseUrl() . '<br/>'; // 获取URL访问的ROOT地址 echo 'root:' . $request->root() . '<br/>'; // 获取URL访问的ROOT地址 echo 'root with domain: ' . $request->root(true) . '<br/>'; // 获取URL地址中的PATH_INFO信息 echo 'pathinfo: ' . $request->pathinfo() . '<br/>'; // 获取URL地址中的PATH_INFO信息 不含后缀 echo 'pathinfo: ' . $request->path() . '<br/>'; // 获取URL地址中的后缀信息 echo 'ext: ' . $request->ext() . '<br/>'; } }
4. Manipulate variables
Get PARAM variable
PARAM variable is a variable acquisition method provided by the framework for automatically identifying GET, POST or PUT requests. It is the system recommended method for obtaining request parameters. The usage is as follows :
The detection, acquisition and security filtering of global input variables can be completed through the Request object~
// 获取当前请求的name变量 Request::instance()->param('name'); // 获取当前请求的所有变量(经过过滤) Request::instance()->param(); // 获取当前请求的所有变量(原始数据) Request::instance()->param(false); // 获取当前请求的所有变量(包含上传文件) Request::instance()->param(true);
//获取REQUEST变量 Request::instance()->request('id'); // 获取某个request变量 Request::instance()->request(); // 获取全部的request变量(经过过滤) Request::instance()->request(false); // 获取全部的request原始变量数据
5. Binding parameters
The default parameter binding method is according to the variable name Bind;
<?php public function read($id) { return 'id ='.$id; } public function archive($year = '2017',$month = '07') { return 'year ='.$year.'$month ='.$month; }
Input URL:
http://localhost/index.php/lian/index/read/id/544
Output:
The parameters for parameter binding according to the variable name must be consistent with the variable name passed in the URL, but the order of the parameters does not need to be consistent
If the above error is reported, the reason for the error is very simple, because when executing the read operation method, the id parameter must be passed in, but the method cannot obtain the correct id parameter information from the URL address. Since we cannot trust any user input, we recommend that you add a default value to the id parameter of the read method
6. Request type
ThinkPHP5.0 uniformly uses the thinkRequest class to handle request types.
Get request type:
public function hq() { // 是否为 GET 请求 if (Request::instance()->isGet()) echo "当前为 GET 请求"; // 是否为 POST 请求 if (Request::instance()->isPost()) echo "当前为 POST 请求"; // 是否为 PUT 请求 if (Request::instance()->isPut()) echo "当前为 PUT 请求"; // 是否为 DELETE 请求 if (Request::instance()->isDelete()) echo "当前为 DELETE 请求"; // 是否为 Ajax 请求 if (Request::instance()->isAjax()) echo "当前为 Ajax 请求"; // 是否为 Pjax 请求 if (Request::instance()->isPjax()) echo "当前为 Pjax 请求"; // 是否为手机访问 if (Request::instance()->isMobile()) echo "当前为手机访问"; // 是否为 HEAD 请求 if (Request::instance()->isHead()) echo "当前为 HEAD 请求"; // 是否为 Patch 请求 if (Request::instance()->isPatch()) echo "当前为 PATCH 请求"; // 是否为 OPTIONS 请求 if (Request::instance()->isOptions()) echo "当前为 OPTIONS 请求"; // 是否为 cli if (Request::instance()->isCli()) echo "当前为 cli"; // 是否为 cgi if (Request::instance()->isCgi()) echo "当前为 cgi"; }
The above is the detailed content of Use thinkphp5.0 to obtain information, variables, and binding parameters. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
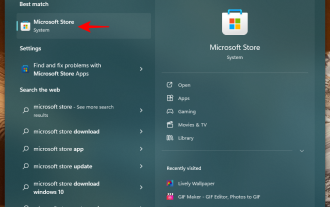
Environment variables are the path to the location (or environment) where applications and programs run. They can be created, edited, managed or deleted by the user and come in handy when managing the behavior of certain processes. Here's how to create a configuration file to manage multiple variables simultaneously without having to edit them individually on Windows. How to use profiles in environment variables Windows 11 and 10 On Windows, there are two sets of environment variables – user variables (apply to the current user) and system variables (apply globally). However, using a tool like PowerToys, you can create a separate configuration file to add new and existing variables and manage them all at once. Here’s how: Step 1: Install PowerToysPowerTo
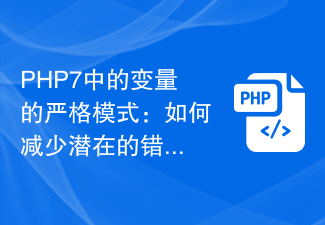
Strict mode was introduced in PHP7, which can help developers reduce potential errors. This article will explain what strict mode is and how to use strict mode in PHP7 to reduce errors. At the same time, the application of strict mode will be demonstrated through code examples. 1. What is strict mode? Strict mode is a feature in PHP7 that can help developers write more standardized code and reduce some common errors. In strict mode, there will be strict restrictions and detection on variable declaration, type checking, function calling, etc. Pass
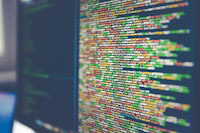
Using Prepared Statements Prepared statements in PDO allow the database to precompile queries and execute them multiple times without recompiling. This is essential to prevent SQL injection attacks, and it can also improve query performance by reducing compilation overhead on the database server. To use prepared statements, follow these steps: $stmt=$pdo->prepare("SELECT*FROMusersWHEREid=?");Bind ParametersBind parameters are a safe and efficient way to provide query parameters that can Prevent SQL injection attacks and improve performance. By binding parameters to placeholders, the database can optimize query execution plans and avoid performing string concatenation. To bind parameters, use the following syntax:
![Internal error: Unable to create temporary directory [Resolved]](https://img.php.cn/upload/article/000/000/164/168171504798267.png?x-oss-process=image/resize,m_fill,h_207,w_330)
Windows system allows users to install various types of applications on your system using executable/setup files. Recently, many Windows users have started complaining that they are receiving an error named INTERNALERROR:cannotCreateTemporaryDirectory on their systems while trying to install any application using an executable file. The problem is not limited to this but also prevents the users from launching any existing applications, which are also installed on the Windows system. Some possible reasons are listed below. Run the executable to install without granting administrator privileges. An invalid or different path was provided for the TMP variable. damaged system
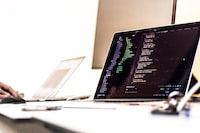
Python is widely used in a wide range of fields with its simple and easy-to-read syntax. It is crucial to master the basic structure of Python syntax, both to improve programming efficiency and to gain a deep understanding of how the code works. To this end, this article provides a comprehensive mind map detailing various aspects of Python syntax. Variables and Data Types Variables are containers used to store data in Python. The mind map shows common Python data types, including integers, floating point numbers, strings, Boolean values, and lists. Each data type has its own characteristics and operation methods. Operators Operators are used to perform various operations on data types. The mind map covers the different operator types in Python, such as arithmetic operators, ratio
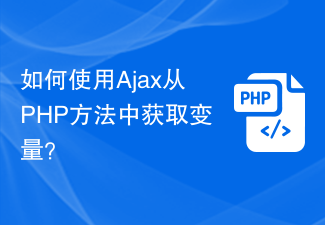
Using Ajax to obtain variables from PHP methods is a common scenario in web development. Through Ajax, the page can be dynamically obtained without refreshing the data. In this article, we will introduce how to use Ajax to get variables from PHP methods, and provide specific code examples. First, we need to write a PHP file to handle the Ajax request and return the required variables. Here is sample code for a simple PHP file getData.php:
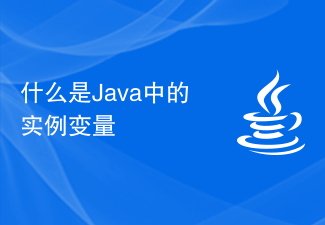
Instance variables in Java refer to variables defined in the class, not in the method or constructor. Instance variables are also called member variables. Each instance of a class has its own copy of the instance variable. Instance variables are initialized during object creation, and their state is saved and maintained throughout the object's lifetime. Instance variable definitions are usually placed at the top of the class and can be declared with any access modifier, which can be public, private, protected, or the default access modifier. It depends on what we want this to be
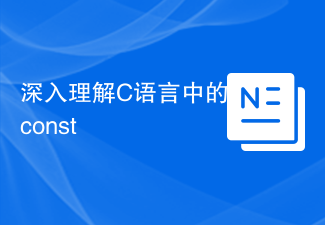
Detailed explanation and code examples of const in C In C language, the const keyword is used to define constants, which means that the value of the variable cannot be modified during program execution. The const keyword can be used to modify variables, function parameters, and function return values. This article will provide a detailed analysis of the use of the const keyword in C language and provide specific code examples. const modified variable When const is used to modify a variable, it means that the variable is a read-only variable and cannot be modified once it is assigned a value. For example: constint
