Parse PHP data type array (Array)
Array in PHP is actually an ordered map. A map is a type that associates values to keys. This type has been optimized in many ways, so it can be treated as a real array, or list (vector), hash table (an implementation of mapping), dictionary, set, stack, queue and more Many possibilities. Since the value of an array element can also be another array, tree structures and multidimensional arrays are also allowed.
Explaining these structures is beyond the scope of this manual, but at least one example will be provided for each structure. For more information on these structures, it is recommended to consult other works on this broad topic.
Syntax
Define array array()
You can use the array() language structure to create a new array. It accepts any number of comma-separated key => value pairs.
array( key => value
, ...
)
// The key can be an integer or a string string
// The value ) can be a value of any type
The comma after the last array element can be omitted. Usually used in single-line array definitions, such as array(1, 2) instead of array(1, 2, ). It is common to leave the last comma in multi-line array definitions to make it easier to add a new cell.
Since 5.4, you can use short array definition syntax, using [] instead of array().
Example #1 A simple array
<?php $array = array( "foo" => "bar", "bar" => "foo", ); // 自 PHP 5.4 起 $array = [ "foo" => "bar", "bar" => "foo", ]; ?>
key can be integer or string. value can be of any type.
In addition, key will have the following forced conversion:
Strings containing legal integer values will be converted to integers. For example, the key name "8" will actually be stored as 8. But "08" will not be cast because it is not a legal decimal value.
Floating point numbers will also be converted to integers, which means their decimal parts will be rounded off. For example, the key name 8.7 will actually be stored as 8.
Boolean values will also be converted to integers. That is, the key name true will actually be stored as 1 and the key name false will be stored as 0.
Null will be converted to an empty string, that is, the key name null will actually be stored as "".
Arrays and objects cannot be used as keys. Insisting on doing this will result in a warning: Illegal offset type.
If multiple units use the same key name in the array definition, only the last one will be used, and the previous ones will be overwritten.
Example #2 Type coercion and overwriting example
<?php $array = array( 1 => "a", "1" => "b", 1.5 => "c", true => "d", ); var_dump($array); ?>
The above routine will output:
array(1) {
[1]=>
string(1) "d"
}
In the above example, all key names are forced to 1, then each new unit will overwrite the previous value, and only one will be left." d".
PHP arrays can contain both integer and string type key names, because PHP does not actually distinguish between index arrays and associative arrays.
If no key name is specified for the given value, the current largest integer index value will be taken, and the new key name will be that value plus one. If the specified key name already has a value, the value will be overwritten.
Example #3 Mixing integer and string key names
<?php $array = array( "foo" => "bar", "bar" => "foo", 100 => -100, -100 => 100, ); var_dump($array); ?>
The above routine will output:
array(4) {
["foo"]=>
string(3) "bar"
["bar"]=>
string(3) "foo"
[100]=>
int(-100)
[-100]=>
int(100)
}
key is optional. If not specified, PHP will automatically use the largest previously used integer key plus 1 as the new key.
Example #4 Index array without key name
<?php $array = array("foo", "bar", "hallo", "world"); var_dump($array); ?>
The above routine will output:
array(4) {
[0]=>
string(3) "foo"
[1]=>
string(3) "bar"
[2]=>
string(5) "hallo"
[ 3]=>
string(5) "world"
}
You can also specify key names only for some units and leave others blank:
Example #5 Only specify key names for some units
<?php $array = array( "a", "b", 6 => "c", "d", ); var_dump($array); ?>
The above routine will output:
array(4) {
[0]=>
string(1) "a"
[1]=>
string(1) "b"
[6]=>
string(1) "c"
[7]=>
string(1) "d"
}
You can see that the last value "d" is automatically assigned the key name 7. This is because the previous largest integer key was 6.
Use square bracket syntax to access array units
Array units can be accessed through array[key] syntax.
Example #6 Accessing the array unit
<?php $array = array( "foo" => "bar", 42 => 24, "multi" => array( "dimensional" => array( "array" => "foo" ) ) ); var_dump($array["foo"]); var_dump($array[42]); var_dump($array["multi"]["dimensional"]["array"]); ?>
The above routine will output:
string(3) "bar"
int(24)
string( 3) "foo"
Note:
Square brackets and curly braces can be used interchangeably to access array units (for example, $array[42] and $array{42} have the effect in the above example same).
自 PHP 5.4 起可以用数组间接引用函数或方法调用的结果。之前只能通过一个临时变量。
自 PHP 5.5 起可以用数组间接引用一个数组原型。
Example #7 数组间接引用
<?php function getArray() { return array(1, 2, 3); } // on PHP 5.4 $secondElement = getArray()[1]; // previously $tmp = getArray(); $secondElement = $tmp[1]; // or list(, $secondElement) = getArray(); ?>
Note:
试图访问一个未定义的数组键名与访问任何未定义变量一样:会导致 E_NOTICE 级别错误信息,其结果为 NULL。
用方括号的语法新建/修改
可以通过明示地设定其中的值来修改一个已有数组。
这是通过在方括号内指定键名来给数组赋值实现的。也可以省略键名,在这种情况下给变量名加上一对空的方括号([])。
$arr[key] = value;
$arr[] = value;
// key 可以是 integer 或 string
// value 可以是任意类型的值
如果 $arr 还不存在,将会新建一个,这也是另一种新建数组的方法。不过并不鼓励这样做,因为如果 $arr 已经包含有值(例如来自请求变量的 string)则此值会保留而 [] 实际上代表着字符串访问运算符。初始化变量的最好方式是直接给其赋值。。
要修改某个值,通过其键名给该单元赋一个新值。要删除某键值对,对其调用 unset() 函数。
<?php $arr = array(5 => 1, 12 => 2); $arr[] = 56; // This is the same as $arr[13] = 56; // at this point of the script $arr["x"] = 42; // This adds a new element to // the array with key "x" unset($arr[5]); // This removes the element from the array unset($arr); // This deletes the whole array ?>
Note:
如上所述,如果给出方括号但没有指定键名,则取当前最大整数索引值,新的键名将是该值加上 1(但是最小为 0)。如果当前还没有整数索引,则键名将为 0。
注意这里所使用的最大整数键名不一定当前就在数组中。它只要在上次数组重新生成索引后曾经存在过就行了。以下面的例子来说明:
<?php // 创建一个简单的数组 $array = array(1, 2, 3, 4, 5); print_r($array); // 现在删除其中的所有元素,但保持数组本身不变: foreach ($array as $i => $value) { unset($array[$i]); } print_r($array); // 添加一个单元(注意新的键名是 5,而不是你可能以为的 0) $array[] = 6; print_r($array); // 重新索引: $array = array_values($array); $array[] = 7; print_r($array); ?>
以上例程会输出:
Array
(
[0] => 1
[1] => 2
[2] => 3
[3] => 4
[4] => 5
)
Array
(
)
Array
(
[5] => 6
)
Array
(
[0] => 6
[1] => 7
)
实用函数
有很多操作数组的函数,参见数组函数一节。
Note:
unset() 函数允许删除数组中的某个键。但要注意数组将不会重建索引。如果需要删除后重建索引,可以用 array_values() 函数。
<?php $a = array(1 => 'one', 2 => 'two', 3 => 'three'); unset($a[2]); /* will produce an array that would have been defined as $a = array(1 => 'one', 3 => 'three'); and NOT $a = array(1 => 'one', 2 =>'three'); */ $b = array_values($a); // Now $b is array(0 => 'one', 1 =>'three') ?>
foreach 控制结构是专门用于数组的。它提供了一个简单的方法来遍历数组。
数组做什么和不做什么
为什么 $foo[bar] 错了?
应该始终在用字符串表示的数组索引上加上引号。例如用 $foo['bar'] 而不是 $foo[bar]。但是为什么呢?可能在老的脚本中见过如下语法:
<?php $foo[bar] = 'enemy'; echo $foo[bar]; // etc ?>
这样是错的,但可以正常运行。那么为什么错了呢?原因是此代码中有一个未定义的常量(bar)而不是字符串('bar'-注意引号),而 PHP 可能会在以后定义此常量,不幸的是你的代码中有同样的名字。它能运行,是因为 PHP 自动将裸字符串(没有引号的字符串且不对应于任何已知符号)转换成一个其值为该裸字符串的正常字符串。例如,如果没有常量定义为 bar,PHP 将把它替代为 'bar' 并使用之。
Note: 这并不意味着总是给键名加上引号。用不着给键名为常量或变量的加上引号,否则会使 PHP 不能解析它们。
<?php error_reporting(E_ALL); ini_set('display_errors', true); ini_set('html_errors', false); // Simple array: $array = array(1, 2); $count = count($array); for ($i = 0; $i < $count; $i++) { echo "\nChecking $i: \n"; echo "Bad: " . $array['$i'] . "\n"; echo "Good: " . $array[$i] . "\n"; echo "Bad: {$array['$i']}\n"; echo "Good: {$array[$i]}\n"; } ?>
以上例程会输出:
Checking 0:
Notice: Undefined index: $i in /path/to/script.html on line 9
Bad:
Good: 1
Notice: Undefined index: $i in /path/to/script.html on line 11
Bad:
Good: 1
Checking 1:
Notice: Undefined index: $i in /path/to/script.html on line 9
Bad:
Good: 2
Notice: Undefined index: $i in /path/to/script.html on line 11
Bad:
Good: 2
演示此行为的更多例子:
<?php // Show all errors error_reporting(E_ALL); $arr = array('fruit' => 'apple', 'veggie' => 'carrot'); // Correct print $arr['fruit']; // apple print $arr['veggie']; // carrot // Incorrect. This works but also throws a PHP error of level E_NOTICE because // of an undefined constant named fruit // // Notice: Use of undefined constant fruit - assumed 'fruit' in... print $arr[fruit]; // apple // This defines a constant to demonstrate what's going on. The value 'veggie' // is assigned to a constant named fruit. define('fruit', 'veggie'); // Notice the difference now print $arr['fruit']; // apple print $arr[fruit]; // carrot // The following is okay, as it's inside a string. Constants are not looked for // within strings, so no E_NOTICE occurs here print "Hello $arr[fruit]"; // Hello apple // With one exception: braces surrounding arrays within strings allows constants // to be interpreted print "Hello {$arr[fruit]}"; // Hello carrot print "Hello {$arr['fruit']}"; // Hello apple // This will not work, and will result in a parse error, such as: // Parse error: parse error, expecting T_STRING' or T_VARIABLE' or T_NUM_STRING' // This of course applies to using superglobals in strings as well print "Hello $arr['fruit']"; print "Hello $_GET['foo']"; // Concatenation is another option print "Hello " . $arr['fruit']; // Hello apple ?>
当打开 error_reporting 来显示 E_NOTICE 级别的错误(将其设为 E_ALL)时将看到这些错误。默认情况下 error_reporting 被关闭不显示这些。
和在语法一节中规定的一样,在方括号(“[”和“]”)之间必须有一个表达式。这意味着可以这样写:
<?php echo $arr[somefunc($bar)]; ?>
这是一个用函数返回值作为数组索引的例子。PHP 也可以用已知常量,可能之前已经见过:
<?php $error_descriptions[E_ERROR] = "A fatal error has occured"; $error_descriptions[E_WARNING] = "PHP issued a warning"; $error_descriptions[E_NOTICE] = "This is just an informal notice"; ?>
注意 E_ERROR 也是个合法的标识符,就和第一个例子中的 bar 一样。但是上一个例子实际上和如下写法是一样的:
<?php $error_descriptions[1] = "A fatal error has occured"; $error_descriptions[2] = "PHP issued a warning"; $error_descriptions[8] = "This is just an informal notice"; ?>
因为 E_ERROR 等于 1,等等。
那么为什么这样做不好?
也许有一天,PHP 开发小组可能会想新增一个常量或者关键字,或者用户可能希望以后在自己的程序中引入新的常量,那就有麻烦了。例如已经不能这样用 empty 和 default 这两个词了,因为他们是保留字。
Note: 重申一次,在双引号字符串中,不给索引加上引号是合法的因此 "$foo[bar]" 是合法的(“合法”的原文为 valid。在实际测试中,这么做确实可以访问数组的该元素,但是会报一个常量未定义的 notice。无论如何,强烈建议不要使用 $foo[bar]这样的写法,而要使用 $foo['bar'] 来访问数组中元素。--haohappy 注)。至于为什么参见以上的例子和字符串中的变量解析中的解释。
转换为数组
对于任意 integer,float,string,boolean 和 resource 类型,如果将一个值转换为数组,将得到一个仅有一个元素的数组,其下标为 0,该元素即为此标量的值。换句话说,(array)$scalarValue 与 array($scalarValue) 完全一样。
如果一个 object 类型转换为 array,则结果为一个数组,其单元为该对象的属性。键名将为成员变量名,不过有几点例外:整数属性不可访问;私有变量前会加上类名作前缀;保护变量前会加上一个 '*' 做前缀。这些前缀的前后都各有一个 NULL 字符。这会导致一些不可预知的行为:
<?php class A { private $A; // This will become '\0A\0A' } class B extends A { private $A; // This will become '\0B\0A' public $AA; // This will become 'AA' } var_dump((array) new B()); ?>
上例会有两个键名为 'AA',不过其中一个实际上是 '\0A\0A'。
将 NULL 转换为 array 会得到一个空的数组。
比较
可以用 array_diff() 和数组运算符来比较数组。
示例
PHP 中的数组类型有非常多的用途。以下是一些示例:
<?php // This: $a = array( 'color' => 'red', 'taste' => 'sweet', 'shape' => 'round', 'name' => 'apple', 4 // key will be 0 ); $b = array('a', 'b', 'c'); // . . .is completely equivalent with this: $a = array(); $a['color'] = 'red'; $a['taste'] = 'sweet'; $a['shape'] = 'round'; $a['name'] = 'apple'; $a[] = 4; // key will be 0 $b = array(); $b[] = 'a'; $b[] = 'b'; $b[] = 'c'; // After the above code is executed, $a will be the array // array('color' => 'red', 'taste' => 'sweet', 'shape' => 'round', // 'name' => 'apple', 0 => 4), and $b will be the array // array(0 => 'a', 1 => 'b', 2 => 'c'), or simply array('a', 'b', 'c'). ?>
Example #8 使用 array()
<?php // Array as (property-)map $map = array( 'version' => 4, 'OS' => 'Linux', 'lang' => 'english', 'short_tags' => true ); // strictly numerical keys $array = array( 7, 8, 0, 156, -10 ); // this is the same as array(0 => 7, 1 => 8, ...) $switching = array( 10, // key = 0 5 => 6, 3 => 7, 'a' => 4, 11, // key = 6 (maximum of integer-indices was 5) '8' => 2, // key = 8 (integer!) '02' => 77, // key = '02' 0 => 12 // the value 10 will be overwritten by 12 ); // empty array $empty = array(); ?>
Example #9 集合
<?php $colors = array('red', 'blue', 'green', 'yellow'); foreach ($colors as $color) { echo "Do you like $color?\n"; } ?>
以上例程会输出:
Do you like red?
Do you like blue?
Do you like green?
Do you like yellow?
直接改变数组的值自 PHP 5 起可以通过引用传递来做到。之前的版本需要需要采取变通的方法:
Example #10 在循环中改变单元
<?php // PHP 5 foreach ($colors as &$color) { $color = strtoupper($color); } unset($color); /* ensure that following writes to $color will not modify the last array element */ // Workaround for older versions foreach ($colors as $key => $color) { $colors[$key] = strtoupper($color); } print_r($colors); ?>
以上例程会输出:
Array
(
[0] => RED
[1] => BLUE
[2] => GREEN
[3] => YELLOW
)
本例生成一个下标从 1 开始的数组。
Example #11 下标从 1 开始的数组
<?php $firstquarter = array(1 => 'January', 'February', 'March'); print_r($firstquarter); ?>
以上例程会输出:
Array
(
[1] => 'January'
[2] => 'February'
[3] => 'March'
)
Example #12 填充数组
<?php // fill an array with all items from a directory $handle = opendir('.'); while (false !== ($file = readdir($handle))) { $files[] = $file; } closedir($handle); ?>
数组是有序的。也可以使用不同的排序函数来改变顺序。更多信息参见数组函数。可以用 count() 函数来数出数组中元素的个数。
Example #13 数组排序
<?php sort($files); print_r($files); ?>
因为数组中的值可以为任意值,也可是另一个数组。这样可以产生递归或多维数组。
Example #14 递归和多维数组
<?php $fruits = array ( "fruits" => array ( "a" => "orange", "b" => "banana", "c" => "apple" ), "numbers" => array ( 1, 2, 3, 4, 5, 6 ), "holes" => array ( "first", 5 => "second", "third" ) ); // Some examples to address values in the array above echo $fruits["holes"][5]; // prints "second" echo $fruits["fruits"]["a"]; // prints "orange" unset($fruits["holes"][0]); // remove "first" // Create a new multi-dimensional array $juices["apple"]["green"] = "good"; ?>
数组(Array) 的赋值总是会涉及到值的拷贝。使用引用运算符通过引用来拷贝数组。
<?php $arr1 = array(2, 3); $arr2 = $arr1; $arr2[] = 4; // $arr2 is changed, // $arr1 is still array(2, 3) $arr3 = &$arr1; $arr3[] = 4; // now $arr1 and $arr3 are the same ?>
The above is the detailed content of Parse PHP data type array (Array). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
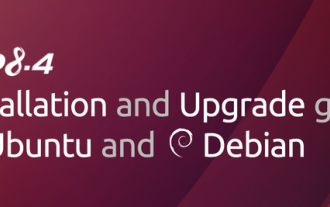
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
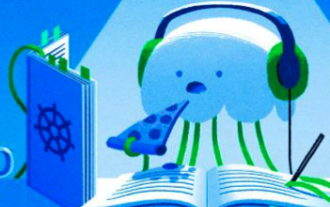
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
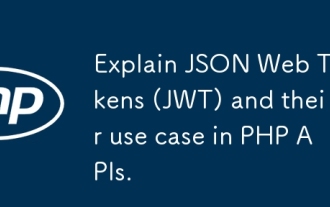
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
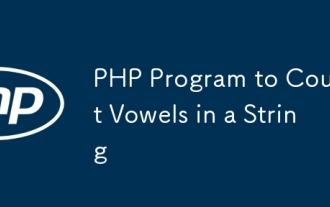
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
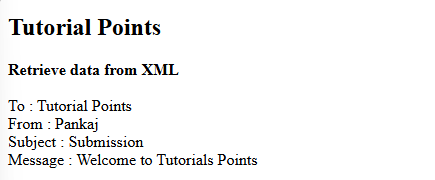
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
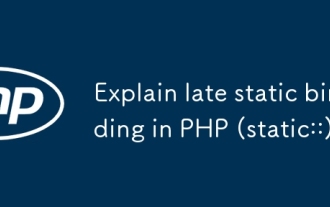
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
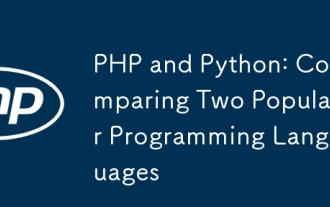
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
