A brief analysis of JavaScript error handling and stack tracing
Sometimes in the process of our code accumulation, some details of error handling and stack traces are ignored, but if you pay attention to these details, they are very useful for writing libraries related to testing or error handling. Now I will give you a great processing idea. This idea can greatly improve the way we handle the stack. When the user's assertion fails, we will give more prompt information (to help the user locate).
Properly handling stack information allows you to clear useless data and focus only on useful data. At the same time, a better understanding of the Errors object and its related properties can help you make fuller use
Errors.
How the call stack (function) works
Before talking about errors, we must first understand the principle of the call stack (function):
When a function is called, it is pushed to the top of the stack. After the function is completed, it will be removed from the top of the stack.
The data structure of the stack is backward First out, known as LIFO (last in, first out).
For example:
function c() { console.log('c'); } function b() { console.log('b'); c(); } function a() { console.log('a'); b(); } a();
In the above example, when function a runs , which will be added to the top of the stack. Then, when function b is called inside function a, function b will be pushed to the top of the stack. When function c is called inside function b It will also be pushed to the top of the stack when called.
When function c runs, the stack contains a, b and c (in this order).
When function c runs After completion, it will be removed from the top of the stack, and then the control flow of the function call returns to function b. After function b finishes running, it will also be removed from the top of the stack, and then the function call
The control flow returns to function a. Finally, function a will be removed from the top of the stack after it is completed.
In order to better demonstrate the behavior of the stack in the demo, you can use console.trace( ) Output the current stack data on the console. At the same time, you have to read the output stack data in order from top to bottom.
function c() { console.log('c'); console.trace(); } function b() { console.log('b'); c(); } function a() { console.log('a'); b(); } a();
Running the above code in Node's REPL mode will get the following output:
Trace at c (repl:3:9) at b (repl:3:1) at a (repl:3:1) at repl:1:1 // <-- For now feel free to ignore anything below this point, these are Node's internals at realRunInThisContextScript (vm.js:22:35) at sigintHandlersWrap (vm.js:98:12) at ContextifyScript.Script.runInThisContext (vm.js:24:12) at REPLServer.defaultEval (repl.js:313:29) at bound (domain.js:280:14) at REPLServer.runBound [as eval] (domain.js:293:12)
As you can see, when output from function c, the stack contains functions a, b and c.
If running in function c After completion, output the current stack data in function b, and you will see that function c has been removed from the top of the stack. At this time, the stack only includes functions a and b.
function c() { console.log('c'); } function b() { console.log('b'); c(); console.trace(); } function a() { console.log('a'); b(); }
As you can see , after function c is completed, it has been removed from the top of the stack.
Trace at b (repl:4:9) at a (repl:3:1) at repl:1:1 // <-- For now feel free to ignore anything below this point, these are Node's internals at realRunInThisContextScript (vm.js:22:35) at sigintHandlersWrap (vm.js:98:12) at ContextifyScript.Script.runInThisContext (vm.js:24:12) at REPLServer.defaultEval (repl.js:313:29) at bound (domain.js:280:14) at REPLServer.runBound [as eval] (domain.js:293:12) at REPLServer.onLine (repl.js:513:10)
Error object and error handling
When an error occurs during program execution, an Error object is usually thrown. The Error object can be used as the prototype inherited by the user-defined error object.
Error.prototype object contains the following properties:
constructor – the constructor pointing to the instance
message – the error message
name–Error name (type)
The above are the standard properties of Error.prototype. In addition, different operating environments have their specific properties. For example, Node, Firefox, Chrome, In environments like Edge, IE 10+, Opera and Safari 6+
, the Error object has a stack attribute, which contains the stack trace of the error. The stack trace of an error instance includes the stack trace since the constructor. All stack structures.
If you want to know more about the specific properties of the Error object, you can read this article on MDN.
In order to throw an error, the throw keyword must be used. To catch a thrown error, try...catch must be used to contain the code that may cause the error. The parameter of Catch is the error instance that was thrown.
Like Java, JavaScript also allows try/catch Then use the finally keyword. After handling the error, you can do some cleanup work in the finally statement block.
In terms of syntax, you can use the try statement block without following it with the catch statement block, but it must be followed by finally block. This means there are three different try statement forms:
try...catch
try...finally
try...catch...finally
You can also embed the try statement in the Try statement:
try { try { throw new Error('Nested error.'); // The error thrown here will be caught by its own `catch` clause } catch (nestedErr) { console.log('Nested catch'); // This runs } } catch (err) { console.log('This will not run.'); }
It can also be in catch or finally Embed try statement:
try { console.log('The try block is running...'); } finally { try { throw new Error('Error inside finally.'); } catch (err) { console.log('Caught an error inside the finally block.'); } }
It is important to note that when throwing an error, you can just throw a simple value instead of the Error object. Although this looks cool and is allowed, it is not A recommended approach, especially for developers of libraries and frameworks that need to deal with other people's code, because there is no standard to refer to and no way of knowing what to expect from users. You cannot trust users to throw Error objects because they May not do this, but simply throw a string or value. This also means that it is difficult to deal with stack information and other meta-information.
For example:
function runWithoutThrowing(func) { try { func(); } catch (e) { console.log('There was an error, but I will not throw it.'); console.log('The error\'s message was: ' + e.message) } } function funcThatThrowsError() { throw new TypeError('I am a TypeError.'); } runWithoutThrowing(funcThatThrowsError);
If the user The parameter passed to the function runWithoutThrowing throws an error object, and the above code can catch the error normally. Then, if it throws a string, you will encounter some problems:
function runWithoutThrowing(func) { try { func(); } catch (e) { console.log('There was an error, but I will not throw it.'); console.log('The error\'s message was: ' + e.message) } } function funcThatThrowsString() { throw 'I am a String.'; } runWithoutThrowing(funcThatThrowsString);
现在第二个 console.log 会输出undefined. 这看起来不是很重要, 但如果你需要确保 Error 对象有一个特定的属性或者用另一种方式来处理 Error 对象的特定属性(例如 Chai的throws断言的做法), 你就得做大量的工作来确保程序的正确运行.同时, 如果抛出的不是 Error 对象, 也就获取不到 stack 属性.
Errors 也可以被作为其它对象, 你也不必抛出它们, 这也是为什么大多数回调函数把 Errors 作为第一个参数的原因. 例如:
const fs = require('fs'); fs.readdir('/example/i-do-not-exist', function callback(err, dirs) { if (err instanceof Error) { // `readdir` will throw an error because that directory does not exist // We will now be able to use the error object passed by it in our callback function console.log('Error Message: ' + err.message); console.log('See? We can use Errors without using try statements.'); } else { console.log(dirs); } });
最后, Error 对象也可以用于 rejected promise, 这使得很容易处理 rejected promise:
new Promise(function(resolve, reject) { reject(new Error('The promise was rejected.')); }).then(function() { console.log('I am an error.'); }).catch(function(err) { if (err instanceof Error) { console.log('The promise was rejected with an error.'); console.log('Error Message: ' + err.message); } });
处理堆栈
这一节是针对支持 Error.captureStackTrace的运行环境, 例如Nodejs.
Error.captureStackTrace 的第一个参数是 object, 第二个可选参数是一个 function.Error.captureStackTrace 会捕获堆栈信息, 并在第一个参数中创建
stack 属性来存储捕获到的堆栈信息. 如果提供了第二个参数, 该函数将作为堆栈调用的终点. 因此, 捕获到的堆栈信息将只显示该函数调用之前的信息.
用下面的两个demo来解释一下. 第一个, 仅将捕获到的堆栈信息存于一个普通的对象之中:
const myObj = {}; function c() { } function b() { // Here we will store the current stack trace into myObj Error.captureStackTrace(myObj); c(); } function a() { b(); } // First we will call these functions a(); // Now let's see what is the stack trace stored into myObj.stack console.log(myObj.stack); // This will print the following stack to the console: // at b (repl:3:7) <-- Since it was called inside B, the B call is the last entry in the stack // at a (repl:2:1) // at repl:1:1 <-- Node internals below this line // at realRunInThisContextScript (vm.js:22:35) // at sigintHandlersWrap (vm.js:98:12) // at ContextifyScript.Script.runInThisContext (vm.js:24:12) // at REPLServer.defaultEval (repl.js:313:29) // at bound (domain.js:280:14) // at REPLServer.runBound [as eval] (domain.js:293:12) // at REPLServer.onLine (repl.js:513:10)
从上面的示例可以看出, 首先调用函数 a(被压入堆栈), 然后在 a 里面调用函数 b(被压入堆栈且在a之上), 然后在 b 中捕获到当前的堆栈信息, 并将其存储到 myObj 中. 所以, 在控制台输出的堆栈信息中仅包含了 a和 b 的调用信息.
现在, 我们给 Error.captureStackTrace 传递一个函数作为第二个参数, 看下输出信息:
const myObj = {}; function d() { // Here we will store the current stack trace into myObj // This time we will hide all the frames after `b` and `b` itself Error.captureStackTrace(myObj, b); } function c() { d(); } function b() { c(); } function a() { b(); } // First we will call these functions a(); // Now let's see what is the stack trace stored into myObj.stack console.log(myObj.stack); // This will print the following stack to the console: // at a (repl:2:1) <-- As you can see here we only get frames before `b` was called // at repl:1:1 <-- Node internals below this line // at realRunInThisContextScript (vm.js:22:35) // at sigintHandlersWrap (vm.js:98:12) // at ContextifyScript.Script.runInThisContext (vm.js:24:12) // at REPLServer.defaultEval (repl.js:313:29) // at bound (domain.js:280:14) // at REPLServer.runBound [as eval] (domain.js:293:12) // at REPLServer.onLine (repl.js:513:10) // at emitOne (events.js:101:20)
当将函数 b 作为第二个参数传给 Error.captureStackTraceFunction 时, 输出的堆栈就只包含了函数 b 调用之前的信息(尽管 Error.captureStackTraceFunction 是在函数 d 中调用的), 这也就是为什么只在控制台输出了 a. 这样处理方式的好处就是用来隐藏一些与用户无关的内部实现细节.
感谢大家阅读本篇文章,之后也会给大家带来关于JS,关于前端的一些小技巧,希望大家共同探讨,一起进步。
相关推荐:
JavaScript刷新页面location.reload()的用法
The above is the detailed content of A brief analysis of JavaScript error handling and stack tracing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
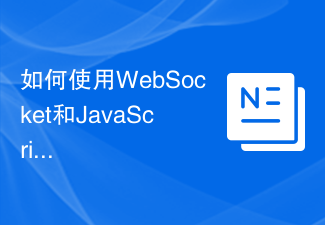
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
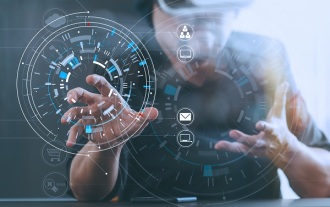
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
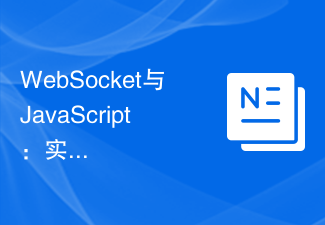
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
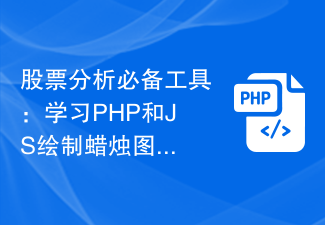
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
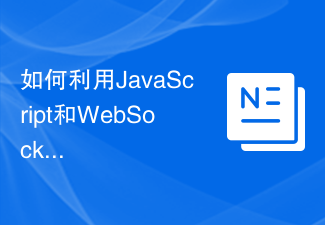
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
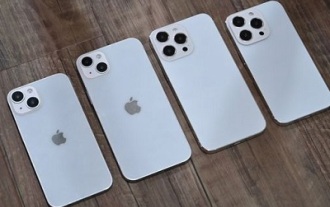
It is possible to recover an Apple phone if it is lost and turned off. The method is also very simple. Users can choose to log in to the official iCloud website to search, or a friend who also uses an Apple phone can use his phone to search for your iPhone. How to track the precise location of an Apple phone if it is lost and turned off? Answer: Search on the official iCloud website or borrow someone else's iPhone device to find it. 1. Users find that their Apple phone is lost or missing, and it can be found even if it is turned off. 2. Users directly log in to the iCloud official website, click Find My iPhone, and be sure to enter the correct account number. 3. Make sure your account is consistent with the account of the lost phone so that you have a chance to recover the phone. 4. If the phone is turned on and connected
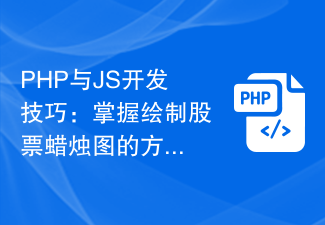
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
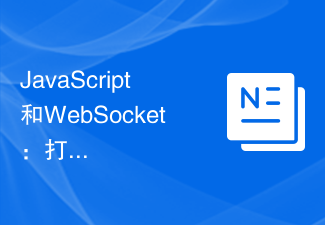
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
