如何使用Python获取给定字符串中的第N个单词?
We can get the Nth Word in a Given String in Python using string splitting, regular expressions, split() method, etc. Manipulating strings is a common task in programming, and extracting specific words from a string can be particularly useful in various scenarios. In this article, we will explore different methods to extract the Nth word from a given string using Python.
方法1:字符串分割
这种方法涉及将字符串分割为单词列表,并根据索引访问所需的单词。
Syntax
words = string.split()
Here, the split() method splits the string based on whitespace by default. The resulting words are stored in the words list.
算法
Accept the input string and the value of N.
使用split()方法将字符串拆分为单词列表。
Access the Nth word from the list using indexing.
Access the Nth word from the list using indexing.
Example
让我们考虑以下字符串:"The quick brown fox jumps over the lazy dog." 在下面的示例中,输入字符串使用split()方法分割成一个单词列表。由于N的值为3,函数返回第三个单词,"brown"。
def get_nth_word_split(string, n): words = string.split() if 1 <= n <= len(words): return words[n-1] else: return "Invalid value of N." # Test the function input_string = "The quick brown fox jumps over the lazy dog." n = 3 print(get_nth_word_split(input_string, n))
输出
brown
方法二:使用正则表达式
Another method to extract the Nth word involves using regular expressions. This approach provides flexibility in handling different word patterns and delimiters.
Syntax
import re words = re.findall(pattern, string)
在这里,使用 re 模块中的 re.findall() 函数根据指定的模式从输入字符串中提取所有单词。模式是使用正则表达式定义的。提取到的单词存储在 words 列表中。
算法
导入 re 模块。
Accept the input string and the value of N.
定义一个正则表达式模式来匹配单词。
Use the findall() function from the re module to extract all words from the string.
Access the Nth word from the list obtained in step 4 using indexing.
返回第N个单词。
Example
在下面的示例中,正则表达式模式'w+'用于匹配输入字符串中的所有单词。findall()函数提取所有单词并将它们存储在一个列表中。由于N的值为4,该函数返回第四个单词"jumps"。
import re def get_nth_word_regex(string, n): pattern = r'\w+' words = re.findall(pattern, string) if 1 <= n <= len(words): return words[n-1] else: return "Invalid value of N." # Test the function input_string = "The quick brown fox jumps over the lazy dog." n = 4 print(get_nth_word_regex(input_string, n))
输出
fox
方法3:使用split()方法和自定义分隔符
In some cases, the string may have a specific delimiter other than whitespace. In such scenarios, we can modify the first method by specifying a custom delimiter for splitting the string.
Syntax
words = string.split(delimiter)
在这里,split()方法被用来根据自定义的分隔符将输入字符串拆分为一个单词列表。分隔符作为参数传递给split()方法。拆分后的单词存储在words列表中。
算法
Accept the input string, the delimiter, and the value of N.
使用split()方法和自定义分隔符将字符串分割成单词列表。
Access the Nth word from the list using indexing
返回第N个单词。
Example
在下面的示例中,字符串使用自定义分隔符(逗号“,”)被分割为单词。第二个单词“banana”被提取并返回,因为它对应于N的值为2。
def get_nth_word_custom_delimiter(string, delimiter, n): words = string.split(delimiter) if 1 <= n <= len(words): return words[n-1] else: return "Invalid value of N." # Test the function input_string = "apple,banana,orange,mango" delimiter = "," n = 2 print(get_nth_word_custom_delimiter(input_string, delimiter, n))
输出
banana
Conclusion
在本文中,我们讨论了如何借助拆分字符串、使用正则表达式以及使用带有自定义分隔符的拆分方法来获取给定字符串中的第n个单词。每种方法都根据任务的要求提供了灵活性。通过理解和实施这些技术,您可以在Python程序中高效地提取字符串中的特定单词。
以上是如何使用Python获取给定字符串中的第N个单词?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
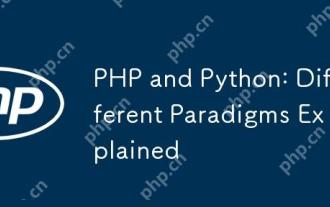
PHP主要是过程式编程,但也支持面向对象编程(OOP);Python支持多种范式,包括OOP、函数式和过程式编程。PHP适合web开发,Python适用于多种应用,如数据分析和机器学习。
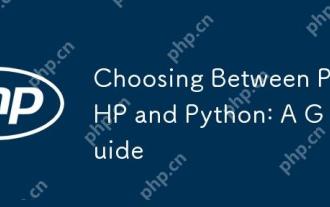
PHP适合网页开发和快速原型开发,Python适用于数据科学和机器学习。1.PHP用于动态网页开发,语法简单,适合快速开发。2.Python语法简洁,适用于多领域,库生态系统强大。
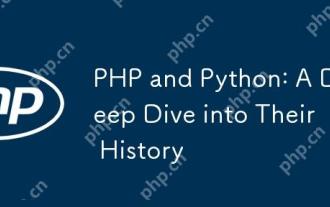
PHP起源于1994年,由RasmusLerdorf开发,最初用于跟踪网站访问者,逐渐演变为服务器端脚本语言,广泛应用于网页开发。Python由GuidovanRossum于1980年代末开发,1991年首次发布,强调代码可读性和简洁性,适用于科学计算、数据分析等领域。
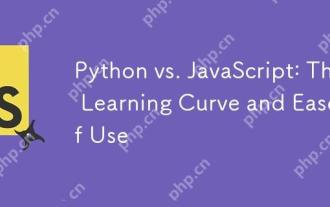
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
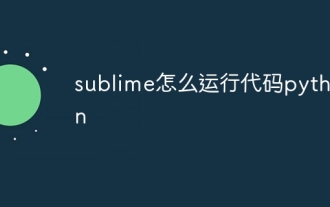
在 Sublime Text 中运行 Python 代码,需先安装 Python 插件,再创建 .py 文件并编写代码,最后按 Ctrl B 运行代码,输出会在控制台中显示。
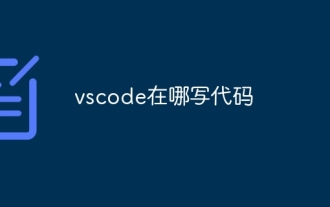
在 Visual Studio Code(VSCode)中编写代码简单易行,只需安装 VSCode、创建项目、选择语言、创建文件、编写代码、保存并运行即可。VSCode 的优点包括跨平台、免费开源、强大功能、扩展丰富,以及轻量快速。
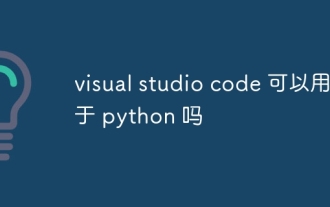
VS Code 可用于编写 Python,并提供许多功能,使其成为开发 Python 应用程序的理想工具。它允许用户:安装 Python 扩展,以获得代码补全、语法高亮和调试等功能。使用调试器逐步跟踪代码,查找和修复错误。集成 Git,进行版本控制。使用代码格式化工具,保持代码一致性。使用 Linting 工具,提前发现潜在问题。
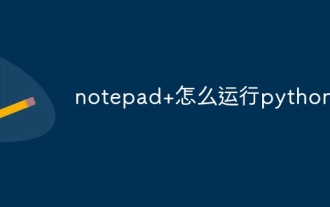
在 Notepad 中运行 Python 代码需要安装 Python 可执行文件和 NppExec 插件。安装 Python 并为其添加 PATH 后,在 NppExec 插件中配置命令为“python”、参数为“{CURRENT_DIRECTORY}{FILE_NAME}”,即可在 Notepad 中通过快捷键“F6”运行 Python 代码。
