Python循环控制:对于vs -a -a比较
在Python中,for循环适用于已知迭代次数的情况,而while循环适合未知迭代次数且需要更多控制的情况。1)for循环适用于遍历序列,如列表、字符串等,代码简洁且Pythonic。2)while循环在需要根据条件控制循环或等待用户输入时更合适,但需注意避免无限循环。3)性能上,for循环略快,但差异通常不大。选择合适的循环类型可以提高代码的效率和可读性。
When it comes to loop control in Python, the age-old debate often boils down to choosing between for
and while
loops. Both have their unique strengths and use cases, and understanding when to use each can significantly improve your coding efficiency and readability. In my journey as a developer, I've seen both loops shine in different scenarios, and I'll share some insights on their differences, use cases, and the nuances that might not be immediately apparent.
Let's dive into the world of Python loops and see how for
and while
stack up against each other.
In Python, for
loops are often used when you know the number of iterations in advance. They're perfect for iterating over sequences like lists, tuples, or strings. Here's a simple example:
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit)
On the other hand, while
loops are more flexible and are used when you don't know the number of iterations beforehand. They continue to run as long as a condition is true. Here's a classic example of a while
loop:
count = 0 while count < 5: print(count) count = 1
Now, let's explore the nuances and use cases of each loop type.
When you're working with sequences, for
loops are not only more readable but also more Pythonic. They automatically handle the iteration, which means less code and fewer chances for errors. For instance, if you want to iterate over a list and perform an action on each item, a for
loop is the go-to choice:
numbers = [1, 2, 3, 4, 5] squared_numbers = [num ** 2 for num in numbers] print(squared_numbers) # Output: [1, 4, 9, 16, 25]
However, for
loops can be less intuitive when you need to break out of the loop early or when the iteration depends on a condition that's not related to the sequence itself. That's where while
loops shine. They give you more control over the loop's execution, allowing you to break out of the loop at any point or continue based on a complex condition.
Consider a scenario where you're waiting for user input and want to keep asking until a valid input is provided:
user_input = "" while user_input.lower() not in ["yes", "no"]: user_input = input("Please enter 'yes' or 'no': ") print(f"You entered: {user_input}")
In this case, a while
loop is more suitable because it allows you to keep asking for input until the condition is met.
One of the common pitfalls I've encountered with while
loops is the potential for infinite loops. If you're not careful with your condition or the way you update the loop variable, you might end up with a loop that never terminates. Here's an example of how to avoid this:
# Incorrect: This will cause an infinite loop # count = 0 # while count < 5: # print(count) # Correct: Make sure to update the loop variable count = 0 while count < 5: print(count) count = 1 # Don't forget this!
Another aspect to consider is performance. In general, for
loops are slightly faster than while
loops because they're optimized for iterating over sequences. However, the difference is usually negligible unless you're dealing with very large datasets. Here's a quick comparison:
import time # Using a for loop start_time = time.time() for i in range(1000000): pass for_loop_time = time.time() - start_time # Using a while loop start_time = time.time() i = 0 while i < 1000000: i = 1 while_loop_time = time.time() - start_time print(f"For loop time: {for_loop_time:.6f} seconds") print(f"While loop time: {while_loop_time:.6f} seconds")
In terms of best practices, it's crucial to choose the right loop for the job. Use for
loops when you're iterating over sequences or when you know the number of iterations in advance. Use while
loops when you need more control over the loop's execution or when the number of iterations is not known beforehand.
From my experience, it's also important to keep your loops as simple and readable as possible. If you find yourself writing complex conditions inside a loop, it might be a sign that you need to refactor your code or consider using a different approach altogether.
In conclusion, both for
and while
loops have their place in Python programming. Understanding their strengths and weaknesses can help you write more efficient and readable code. Whether you're iterating over a list of items or waiting for user input, choosing the right loop can make a significant difference in your code's clarity and performance.
以上是Python循环控制:对于vs -a -a比较的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
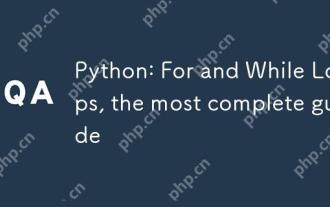
Python中,for循环用于遍历可迭代对象,while循环用于条件满足时重复执行操作。1)for循环示例:遍历列表并打印元素。2)while循环示例:猜数字游戏,直到猜对为止。掌握循环原理和优化技巧可提高代码效率和可靠性。

如何使用Python中的while循环在Python编程中,循环是非常重要的概念之一。循环可以帮助我们重复执行一段代码,直到满足指定条件为止。其中,while循环是一种被广泛使用的循环结构之一。通过使用while循环,我们可以根据条件的真假进行重复执行,从而实现更复杂的逻辑。使用while循环的基本语法格式如下:while条件:循环体其中,条件是
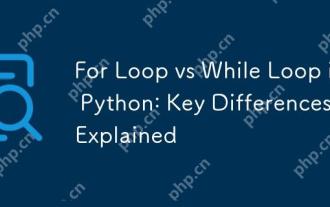
在您的知识之际,而foroopsareideal insinAdvance中,而WhileLoopSareBetterForsituations则youneedtoloopuntilaconditionismet
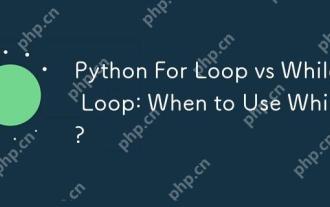
useeAforloopWheniteratingOveraseQuenceOrforAspecificnumberoftimes; useAwhiLeLoopWhenconTinuingUntilAcIntiment.ForloopSareIdeAlforkNownsences,而WhileLeleLeleLeleLoopSituationSituationSituationsItuationSuationSituationswithUndEtermentersitations。

Useforloopswhenthenumberofiterationsisknowninadvance,andwhileloopswheniterationsdependonacondition.1)Forloopsareidealforsequenceslikelistsorranges.2)Whileloopssuitscenarioswheretheloopcontinuesuntilaspecificconditionismet,usefulforuserinputsoralgorit
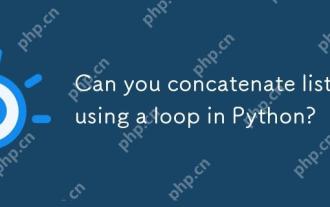
是的,YouCanconCatenatElistsusingAloopInpyThon.1)使用eparateLoopsForeachListToAppendIteMstoaresultList.2)useanestedlooptoiterateOverMultipliplipliplipliplipliplipliplipliplipliplistforamoreConciseApprace.3)
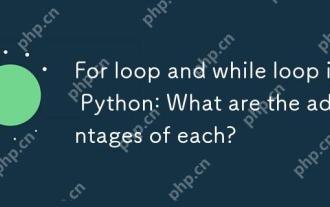
forloopsareadvantageousforknowniterations and sequests,供应模拟性和可读性;而LileLoopSareIdealFordyNamicConcitionSandunknowniterations,提供ControloperRoverTermination.1)forloopsareperfectForeTectForeTerToratingOrtratingRiteratingOrtratingRitterlistlistslists,callings conspass,calplace,cal,ofstrings ofstrings,orstrings,orstrings,orstrings ofcces
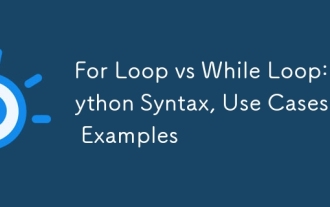
foroopsare whenthenemberofiterationsisknown,而whileLoopsareUseduntilacTitionismet.1)ForloopSareIdealForeSequencesLikeLists,UsingSyntaxLike'forfruitinFruitinFruitinFruitIts:print(fruit)'。2)'
