Mastering JavaScript Proxy and Reflect API (Without the Headache)
Table of Contents
- Introduction
-
What’s the Deal with JavaScript Proxy?
- Real-life Example: Tracking Object Changes (The Simple Way)
-
When You’d Want to Use a Proxy
- Data Validation Done Right
- Building Reactive Objects (Yeah, Vue Does This)
- Lazy Objects: Only Create What You Need
- Okay, But What About Reflect
-
How Proxy and Reflect are the Perfect Duo
- Real-life Example: Logging with Style
- Why You’ll Love Proxy & Reflect
- Wrapping It Up
Introduction ?
Let’s talk about two of probably the most underrated (and underused) features of JavaScript: Proxy and Reflect. I get it — you’ve probably heard of them, maybe even Googled them once or twice, but they seem a little extra, right?
But here’s the thing: these tools can unlock a whole new level of control over your objects, and they’re not as hard to use as you might think. By the end of this post, you’ll know exactly how they work, why they’re awesome, and how to add them to your toolbox for practical, real-world scenarios.
Let’s dive in!
What’s the Deal with JavaScript Proxy?
A Proxy in JavaScript is like the ultimate middleman. It lets you intercept and customize how you interact with objects. You can control what happens when someone tries to access, modify, or delete a property. And the best part? It’s super easy to use.
Think of it this way: you’ve got an object — say, a user profile — and you want to make sure anyone messing with that object doesn’t do anything weird (like setting their age to “gibberish”). With a Proxy, you can jump in and take control.
Here’s the basic syntax:
let proxy = new Proxy(target, handler);
- target: The original object (the one we’re wrapping).
- handler: An object with functions (called traps) that intercept operations like reading, writing, or deleting properties.
Real-life Example: Tracking Object Changes (The Simple Way)
Let’s say you’re building a collaborative editing app, and you want to know every time someone updates the document. Easy enough with a Proxy:
const documentModel = { title: "Draft", content: "Hello World" }; const handler = { set(target, prop, value) { console.log(`Property ${prop} is changing from ${target[prop]} to ${value}`); target[prop] = value; return true; } }; const documentProxy = new Proxy(documentModel, handler); documentProxy.title = "Final Draft"; // Logs: Property title is changing from Draft to Final Draft
Every time the document changes, you get a nice little log showing exactly what happened. No surprises here.
When You’d Want to Use a Proxy
So, when should you actually use a Proxy? Great question. Here are some scenarios where it really shines:
Data Validation Done Right
You can use Proxies to enforce data validation rules. No more invalid data sneaking into your app and causing headaches later.
const person = { name: "", age: 0 }; const handler = { set(target, prop, value) { if (prop === "age" && typeof value !== "number") { throw new TypeError("Age must be a number"); } target[prop] = value; return true; } }; const personProxy = new Proxy(person, handler); personProxy.age = 30; // All good personProxy.age = "thirty"; // Throws an error
Now you’ve got some nice validation that can be extended, and you didn’t have to write a ton of boilerplate code. Nice!
Building Reactive Objects (Yeah, Vue Does This)
If you’ve ever worked with Vue, you’ve already seen Proxies in action. Vue uses Proxies to make data reactive, automatically updating the UI when data changes.
You can use a Proxy to watch objects for changes and react in real-time — perfect for building your own reactive systems or dashboards.
Lazy Objects: Only Create What You Need
You can use a Proxy to defer the creation of expensive objects until they’re actually needed. This is called lazy initialization. Instead of loading all your data up front, you only grab what’s required, when it’s required.
Okay, But What About Reflect?
The Reflect API is like Proxy’s best buddy. While Proxy lets you intercept operations, Reflect gives you tools to work with those operations in a more standardized way. It lets you handle object operations (like setting or getting properties) more cleanly and predictably.
Here’s how you can use Reflect to work with default object behaviors:
const user = { name: "Alice", age: 25 }; console.log(Reflect.get(user, "name")); // Alice Reflect.set(user, "age", 30); // Sets age to 30
Why bother with Reflect? It makes code easier to read and more consistent. You can use it with Proxies to handle default behavior when you don’t want to do anything custom.
How Proxy and Reflect are the Perfect Duo
Let’s put Proxy and Reflect together. If you want to add some logging but still handle object operations normally, Reflect is your friend. Here’s an example where we log when properties are accessed or changed but delegate the actual work to Reflect:
const product = { name: "Laptop", price: 1000 }; const handler = { set(target, prop, value) { console.log(`Setting ${prop} to ${value}`); return Reflect.set(target, prop, value); }, get(target, prop) { console.log(`Getting ${prop}`); return Reflect.get(target, prop); } }; const productProxy = new Proxy(product, handler); productProxy.price = 1200; // Logs: Setting price to 1200 console.log(productProxy.price); // Logs: Getting price, Output: 1200
The best part? We can customize the behavior (logging), but Reflect handles the actual logic of setting and getting properties. This keeps things simple and predictable.
Why You’ll Love Proxy & Reflect
Here’s why these tools are awesome:
- Flexibility: You can control how objects behave, whether you’re adding validation, logging, or lazy loading.
- Powerful Abstractions: With Proxies, you can hide complex logic and make it feel like magic. Combine that with Reflect, and you’ve got control and safety.
- Cleaner Code: Instead of hacking around edge cases or writing tons of conditionals, Proxies allow you to intercept behavior in a clean and reusable way.
- Better DX (Developer Experience): Less boilerplate, fewer surprises, more control. What’s not to love?
Wrapping It Up
And there you have it! Proxies and Reflect may seem a little intimidating at first, but once you get the hang of them, they can dramatically improve your code. Whether you’re validating data, tracking object changes, or just wanting more control over how objects behave, these tools are here to make your life easier.
So go ahead, give them a try! You might just find yourself reaching for Proxy and Reflect in your next project.
以上是Mastering JavaScript Proxy and Reflect API (Without the Headache)的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
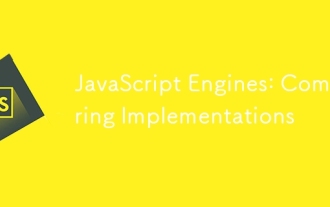
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
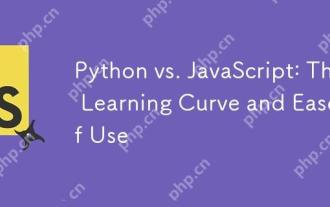
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
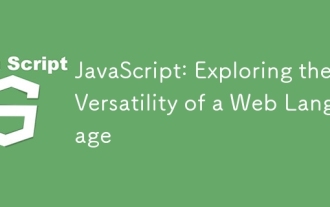
JavaScript是现代Web开发的核心语言,因其多样性和灵活性而广泛应用。1)前端开发:通过DOM操作和现代框架(如React、Vue.js、Angular)构建动态网页和单页面应用。2)服务器端开发:Node.js利用非阻塞I/O模型处理高并发和实时应用。3)移动和桌面应用开发:通过ReactNative和Electron实现跨平台开发,提高开发效率。
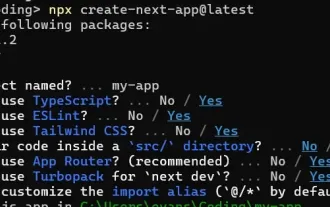
本文展示了与许可证确保的后端的前端集成,并使用Next.js构建功能性Edtech SaaS应用程序。 前端获取用户权限以控制UI的可见性并确保API要求遵守角色库
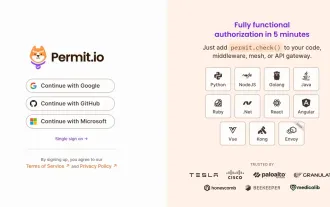
我使用您的日常技术工具构建了功能性的多租户SaaS应用程序(一个Edtech应用程序),您可以做同样的事情。 首先,什么是多租户SaaS应用程序? 多租户SaaS应用程序可让您从唱歌中为多个客户提供服务
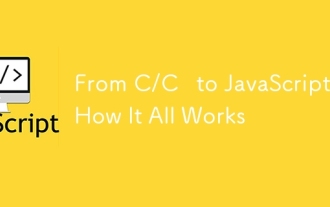
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
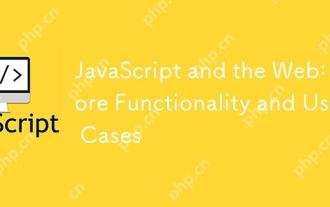
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
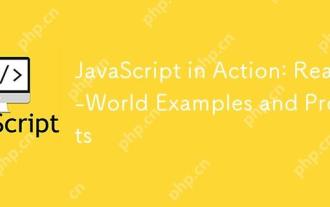
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
