Xor of N numbers
Given an integer number N, find the exor of the range 1 to N
exor of 1 ^ 2 ^ 3 ^4 ^.....N;
Brute force approach:
Tc:O(n)
Sc:O(1)
public int findExor(int N){ //naive/brute force approach: int val = 0; for(int i=1;i<5;i++){ val = val^ i; } return val; }
Optimal approach:
Tc:O(1)
Sc:O(1)
public int getExor(int N){ //better approach /** * one thing to observe is * 1 = 001 = 1 * 1 ^2 = 001 ^ 010 = 011= 3 * 1^2^3 = 011 ^ 011 = 0= 0 * 1^2^3^4 = 000^100 = 100= 4 * 1^2^3^4^5 = 100^101 = 001= 1 * 1^2^3^4^5^6 = 001^110 =111= 7 * 1^2^3^4^5^6^7 = 111^111=000= 0 * * what we can observer is : * * N%4==0 then result is: N * N%4 ==1 then result is: 1 * N%4 ==2 then result is: N+1 * N%4==3 then result is: 0 * * */ if(N%4==0) return N; else if(N%4 ==1) return 1; else if(N%4==2) return N+1; else return 0; }
What if we have to find the exor between ranges like L and R
example find an exor between numbers 4 and 7 i.e. 4^5^6^7.
For solving this we can leverage the same optimal solution above getExor()
first we will get exor till L-1 i.e getExor(L-1) = 1 ^ 2 ^ 3 (since L-1 = 3)......equation(1)
then we will find getExor(R) = 1 ^ 2 ^ 3 ^ 4 ^ 5 ^ 6 ^ 7 ----equation(2)
the finally,
Result = equation(1) ^ equation(2) = (1 ^ 2 ^ 3) ^ (1 ^ 2 ^ 3 ^ 4 ^ 5 ^ 6 ^ 7) = (4^5^6^7)
public int findExorOfRange(int L, int R){ return getExor(L-1) ^ getExor(R); } public int getExor(int N){ //better approach /** * one thing to observe is * 1 = 001 = 1 * 1 ^2 = 001 ^ 010 = 011= 3 * 1^2^3 = 011 ^ 011 = 0= 0 * 1^2^3^4 = 000^100 = 100= 4 * 1^2^3^4^5 = 100^101 = 001= 1 * 1^2^3^4^5^6 = 001^110 =111= 7 * 1^2^3^4^5^6^7 = 111^111=000= 0 * * what we can observer is : * * N%4==0 then result is: N * N%4 ==1 then result is: 1 * N%4 ==2 then result is: N+1 * N%4==3 then result is: 0 * * */ if(N%4==0) return N; else if(N%4 ==1) return 1; else if(N%4==2) return N+1; else return 0; }
以上是Xor of N numbers的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
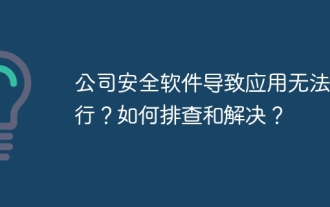
公司安全软件导致部分应用无法正常运行的排查与解决方法许多公司为了保障内部网络安全,会部署安全软件。...
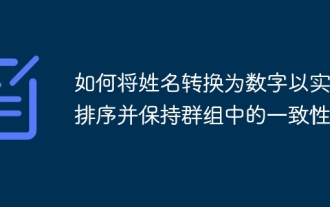
将姓名转换为数字以实现排序的解决方案在许多应用场景中,用户可能需要在群组中进行排序,尤其是在一个用...
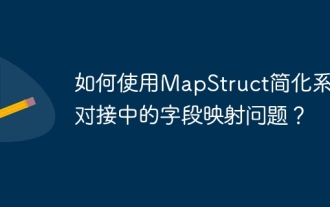
系统对接中的字段映射处理在进行系统对接时,常常会遇到一个棘手的问题:如何将A系统的接口字段有效地映�...
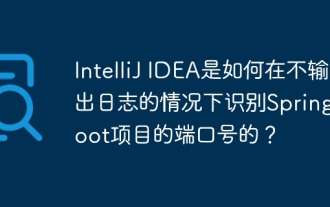
在使用IntelliJIDEAUltimate版本启动Spring...
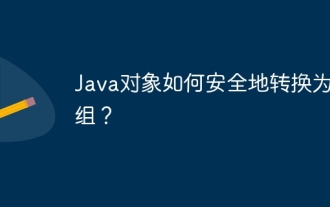
Java对象与数组的转换:深入探讨强制类型转换的风险与正确方法很多Java初学者会遇到将一个对象转换成数组的�...
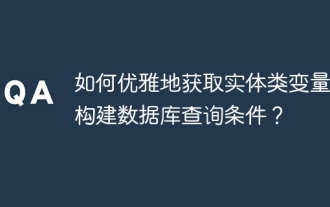
在使用MyBatis-Plus或其他ORM框架进行数据库操作时,经常需要根据实体类的属性名构造查询条件。如果每次都手动...
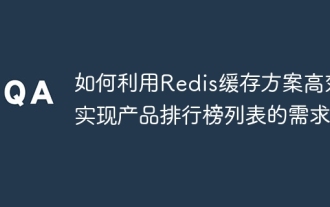
Redis缓存方案如何实现产品排行榜列表的需求?在开发过程中,我们常常需要处理排行榜的需求,例如展示一个�...
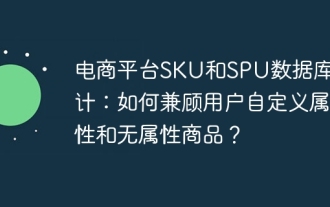
电商平台SKU和SPU表设计详解本文将探讨电商平台中SKU和SPU的数据库设计问题,特别是如何处理用户自定义销售属...
