Colour palette Generator
Hello world ?? I'm back on track. The #100daysofMiva coding challenge day 9 and I worked on a simple colour palette Generator in Nodejs. Let's have a walk through...✨
? Color Palette Generator
Check it out
https://colorpal.onrender.com
This is a Color Palette Generator built with Node.js using Express.js and chroma-js. It generates a beautiful 5-color palette every time, with varying shades of a randomly generated base color. Perfect for UI/UX designers looking for quick color inspiration! ?
? Features
- Generates 5 matching colors as variants of a single base color ?.
- Each palette is assigned a unique ID, allowing users to retrieve it via the /palette/:id route.
- Built with Express.js for server-side logic and chroma-js for color manipulation.
- Simple and elegant UI for displaying the generated palette, with a refresh button to generate new palettes dynamically.
Backend ?
?️ Technologies Used
- Node.js & Express.js: Backend framework to handle routing.
- Chroma.js: For powerful color manipulation and palette generation.
- HTML/CSS/Bootstrap: For a clean, responsive UI.
? Getting Started
1. Clone the Repository
git clone https://github.com/Marvellye/colorpal cd colorpal
2. Install Dependencies
npm install
3. Run the Application
node app.js
4. Open the Application
Visit the app in your browser at:
http://localhost:3000
You will see an interface where you can generate a new 5-color palette. Press the Generate button to fetch a new color palette.
5. Retrieve a Palette by ID
To retrieve a specific palette, go to:
http://localhost:3000/palette/:id
Replace :id with the ID of the palette you want to view, for example:
http://localhost:3000/palette/C08552-F3E9DC-5E3023-DAB49D
? How it Works
- Generating a Palette: Each time you click the Generate button, a base color is randomly generated. From this base, 5 distinct color variants (different shades) are created.
- Unique ID: Each generated palette has a unique ID made from the hexadecimal values of the colors, which can be used to retrieve the palette later.
- Using Chroma.js: The power of chroma-js ensures that the generated colors are visually appealing, offering a variety of brightness levels.
?️ Example Palette
Here’s an example of the kind of palette the app generates:
Base Color: #C08552 Palette: #F3E9DC (Light) #5E3023 (Dark) #DAB49D (Neutral)
Front-end?✨
? Colour Palette display
This Colour Palette Generator dynamically generates a palette of five colors and displays them in the UI. Users can interact with the palette by clicking the Generate button, and the palette ID is used to save and share specific color combinations. Here's how the layout works.
? HTML Layout
Header
<!-- Header --> <header class="p-3 text-center"> <h1>Colour Palette Generator</h1> </header>
The header provides a clean and simple title for the application.
Colour Boxes
<!-- Colour Boxes --> <section class="color-container"> <div id="box1" class="color-box" style="background-color: #000000;"> <span>#000000</span> </div> <div id="box2" class="color-box" style="background-color: #000000;"> <span>#000000</span> </div> <div id="box3" class="color-box" style="background-color: #000000;"> <span>#000000</span> </div> <div id="box4" class="color-box" style="background-color: #000000;"> <span class="text-white">Hey!</span> </div> <div id="box5" class="color-box" style="background-color: #000000;"> <span class="text-white"></span> </div> </section>
This section contains the color boxes. Each box is a div that dynamically changes its background color based on the generated color palette. The span inside each div displays the color's hex value.
Loader
<!-- Loader --> <section id="loader" class="loader"> <div class="is-loading"> <h3 id="load-text">Generating...</h3> </div> </section>
This section contains a loader that appears when a new color palette is being generated. The loader disappears after the colors are loaded.
Footer
<!-- Footer --> <footer class="d-flex justify-content-between align-items-center"> <button onclick="gen()" class="btn btn-light">Generate</button> <button class="btn text-white" onclick="share(window.location.href)"> <i class="fa-regular fa-share-from-square"></i> </button> <span class="">100daysofMiva-Marvelly</span> </footer>
The footer contains a Generate button that triggers the color generation and a share button for social media sharing.
? JavaScript Functionality
Dynamic Color Generation
const boxes = [ document.getElementById('box1'), document.getElementById('box2'), document.getElementById('box3'), document.getElementById('box4'), document.getElementById('box5') ]; function updateBoxes(colors) { colors.forEach((color, index) => { boxes[index].style.backgroundColor = color; boxes[index].querySelector('span').textContent = color; }); }
This JavaScript code dynamically updates the colors in the color boxes when a new palette is generated. The colors are applied to each div element in the UI.
Palette Generation Logic
async function gen() { // Show the loader loader.style.display = 'block'; try { const response = await fetch('/palette'); const data = await response.json(); // Update the boxes with the new colors updateBoxes(data.palette); // Update the URL with the new ID history.pushState({}, '', `/${data.id}`); loader.style.display = 'none'; } catch (error) { console.error('Error fetching palette:', error); loader.style.display = 'none'; } }
The gen() function fetches a new color palette from the /palette API route, updates the color boxes, and modifies the browser URL with the new palette ID.
Share Functionality
function share(url) { Swal.fire({ heightAuto: false, title: 'Share this Color Palette!', html: ` <div style="display: flex; justify-content: space-around; font-size: 24px;"> <a href="https://api.whatsapp.com/send?text=${encodeURIComponent(url)}" target="_blank" title="Share on WhatsApp"> <i class="fab fa-whatsapp" style="color: #25D366;"></i> </a> <a href="https://www.facebook.com/sharer/sharer.php?u=${encodeURIComponent(url)}" target="_blank" title="Share on Facebook"> <i class="fab fa-facebook" style="color: #3b5998;"></i> </a> <a href="https://twitter.com/intent/tweet?url=${encodeURIComponent(url)}" target="_blank" title="Share on Twitter"> <i class="fab fa-twitter" style="color: #1DA1F2;"></i> </a> <a href="https://www.instagram.com/?url=${encodeURIComponent(url)}" target="_blank" title="Share on Instagram"> <i class="fab fa-instagram" style="color: #E1306C;"></i> </a> </div> `, showConfirmButton: false, showCloseButton: false, }); }
The share() function allows users to share the current color palette via social media platforms like WhatsApp, Facebook, Twitter, Instagram, and Telegram using SweetAlert popups.
URL-Based Color Loading
// Check if an id is present in the URL const currentPath = window.location.pathname; const paletteId = currentPath.substring(1); if (paletteId) { loadPaletteById(paletteId); } else { gen(); }
This functionality checks if there is a color palette ID in the URL when the page is loaded. If an ID is present, the corresponding palette is loaded. Otherwise, a new palette is generated.
? Issues Encountered
Building this app wasn't without its challenges! Here are some of the problems I encountered and how I solved them:
Dull Color Palettes: Initially, I was getting dull and boring colors. The issue was due to the way I was generating shades from the base color. I switched to using hsl.l (lightness) adjustments for better visual results.
Color Palette Variants: At first, I used random colors that were too different from each other. After realizing that everyone needed variants of the same base color for consistency, I adjusted my approach to generate color scales with different lightness levels.
Invalid Palette IDs: When trying to retrieve a palette by ID, some IDs were invalid or incorrectly formatted. I fixed this by ensuring a strict format for the IDs and adding error handling for invalid IDs.
Hex Values Misplacement: At one point, the hex values were not displaying properly inside the color boxes. This was fixed by ensuring the span elements were correctly updated with the hex values.
Palette Sharing: Creating a robust sharing mechanism for various social media platforms was a bit challenging but ultimately solved using SweetAlert for the UI and share links for each platform.
Dynamic Palette Update: Implementing a smooth update of the UI when a new palette was generated was tricky. By using simple JavaScript and handling the loader display correctly, I ensured a seamless experience for users when generating new palettes.
✨ Future Improvements
- Add support for saving favorite palettes in a database.
- Enhance the UI with animations for palette generation.
- Allow users to download the color palette in various formats like JSON, CSS, or an image.
Check it out
https://colorpal.onrender.com
My GitHub repo
https://github.com/Marvellye/colorpal
以上是Colour palette Generator的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
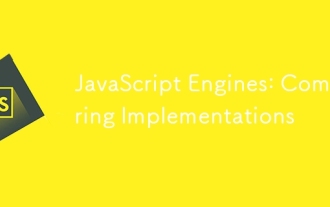
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
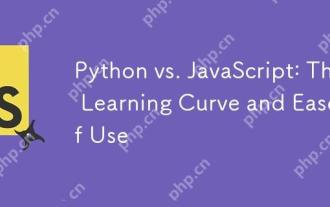
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
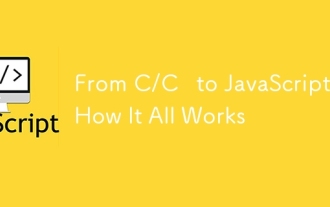
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
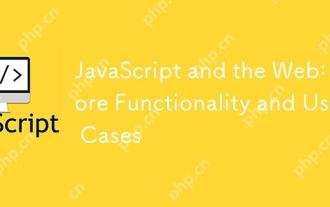
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
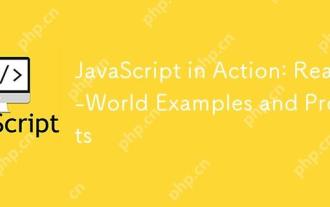
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
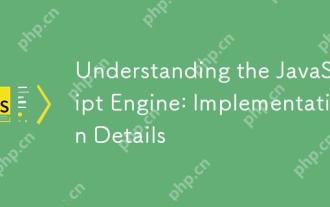
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
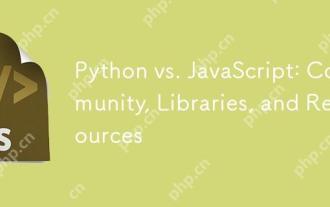
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
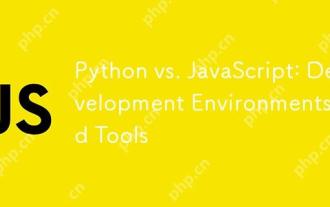
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
