Longest Subarray With Maximum Bitwise AND
2419. Longest Subarray With Maximum Bitwise AND
Difficulty: Medium
Topics: Array, Bit Manipulation, Brainteaser
You are given an integer array nums of size n.
Consider a non-empty subarray from nums that has the maximum possible bitwise AND.
- In other words, let k be the maximum value of the bitwise AND of any subarray of nums. Then, only subarrays with a bitwise AND equal to k should be considered.
Return the length of the longest such subarray.
The bitwise AND of an array is the bitwise AND of all the numbers in it.
A subarray is a contiguous sequence of elements within an array.
Example 1:
- Input: nums = [1,2,3,3,2,2]
- Output: 2
-
Explanation:
- The maximum possible bitwise AND of a subarray is 3.
- The longest subarray with that value is [3,3], so we return 2.
Example 2:
- Input: nums = [1,2,3,4]
- Output: 1
-
Explanation:
- The maximum possible bitwise AND of a subarray is 4.
- The longest subarray with that value is [4], so we return 1.
Constraints:
- 1 <= nums.length <= 101
- 1 <= nums[i] <= 106
Hint:
- Notice that the bitwise AND of two different numbers will always be strictly less than the maximum of those two numbers.
- What does that tell us about the nature of the subarray that we should choose?
Solution:
Let's first break down the problem step by step:
Key Insights:
-
Bitwise AND Properties:
- The bitwise AND of two numbers is generally smaller than or equal to both numbers.
- Therefore, if we find a maximum value in the array, the subarray that will achieve this maximum bitwise AND value must consist of this maximum value repeated.
-
Objective:
- Find the maximum value in the array.
- Find the longest contiguous subarray of that maximum value, because any other number in the subarray would reduce the overall bitwise AND result.
Plan:
- Traverse the array and determine the maximum value.
- Traverse the array again to find the longest contiguous subarray where all elements are equal to this maximum value.
Example:
For the input array [1,2,3,3,2,2], the maximum value is 3. The longest contiguous subarray with only 3s is [3,3], which has a length of 2.
Let's implement this solution in PHP: 2419. Longest Subarray With Maximum Bitwise AND
Explanation:
- Step 1: We first find the maximum value in the array using PHP's built-in max() function.
- Step 2: We initialize two variables, $maxLength to store the length of the longest subarray and $currentLength to track the length of the current contiguous subarray of the maximum value.
- Step 3: We iterate through the array:
- If the current number equals the maximum value, we increment the length of the current subarray.
- If the current number does not equal the maximum value, we check if the current subarray is the longest so far and reset the length.
- Final Step: After the loop, we ensure that if the longest subarray is at the end of the array, we still consider it.
- Finally, we return the length of the longest subarray that contains only the maximum value.
Time Complexity:
- Finding the maximum value takes (O(n)).
- Traversing the array to find the longest subarray takes (O(n)).
- Overall time complexity: (O(n)), where (n) is the length of the array.
Test Cases:
For the input [1, 2, 3, 3, 2, 2], the output is 2, and for [1, 2, 3, 4], the output is 1, as expected.
This solution handles the constraints and efficiently solves the problem.
Contact Links
If you found this series helpful, please consider giving the repository a star on GitHub or sharing the post on your favorite social networks ?. Your support would mean a lot to me!
If you want more helpful content like this, feel free to follow me:
- GitHub
以上是Longest Subarray With Maximum Bitwise AND的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
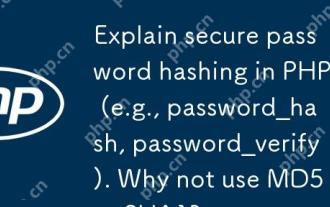
在PHP中,应使用password_hash和password_verify函数实现安全的密码哈希处理,不应使用MD5或SHA1。1)password_hash生成包含盐值的哈希,增强安全性。2)password_verify验证密码,通过比较哈希值确保安全。3)MD5和SHA1易受攻击且缺乏盐值,不适合现代密码安全。
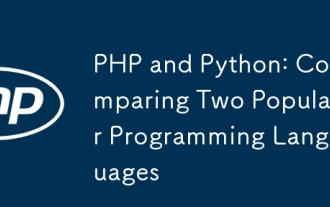
PHP和Python各有优势,选择依据项目需求。1.PHP适合web开发,尤其快速开发和维护网站。2.Python适用于数据科学、机器学习和人工智能,语法简洁,适合初学者。
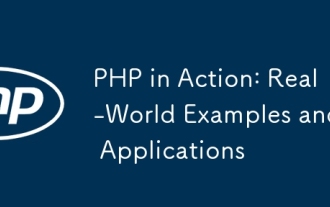
PHP在电子商务、内容管理系统和API开发中广泛应用。1)电子商务:用于购物车功能和支付处理。2)内容管理系统:用于动态内容生成和用户管理。3)API开发:用于RESTfulAPI开发和API安全性。通过性能优化和最佳实践,PHP应用的效率和可维护性得以提升。
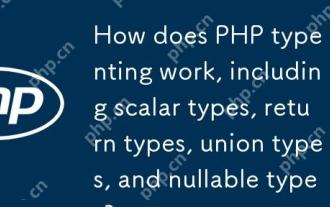
PHP类型提示提升代码质量和可读性。1)标量类型提示:自PHP7.0起,允许在函数参数中指定基本数据类型,如int、float等。2)返回类型提示:确保函数返回值类型的一致性。3)联合类型提示:自PHP8.0起,允许在函数参数或返回值中指定多个类型。4)可空类型提示:允许包含null值,处理可能返回空值的函数。
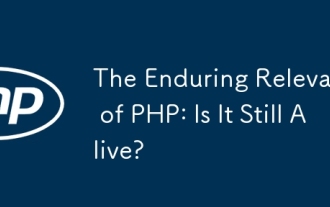
PHP仍然具有活力,其在现代编程领域中依然占据重要地位。1)PHP的简单易学和强大社区支持使其在Web开发中广泛应用;2)其灵活性和稳定性使其在处理Web表单、数据库操作和文件处理等方面表现出色;3)PHP不断进化和优化,适用于初学者和经验丰富的开发者。
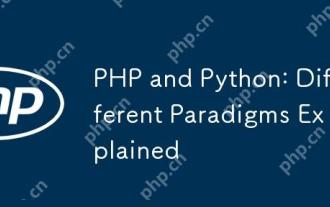
PHP主要是过程式编程,但也支持面向对象编程(OOP);Python支持多种范式,包括OOP、函数式和过程式编程。PHP适合web开发,Python适用于多种应用,如数据分析和机器学习。
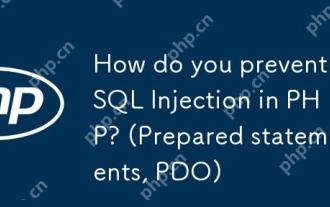
在PHP中使用预处理语句和PDO可以有效防范SQL注入攻击。1)使用PDO连接数据库并设置错误模式。2)通过prepare方法创建预处理语句,使用占位符和execute方法传递数据。3)处理查询结果并确保代码的安全性和性能。
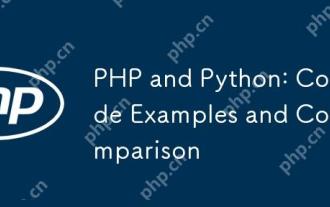
PHP和Python各有优劣,选择取决于项目需求和个人偏好。1.PHP适合快速开发和维护大型Web应用。2.Python在数据科学和机器学习领域占据主导地位。
