如何使用Python獲取給定字串中的第N個單字?
We can get the Nth Word in a Given String in Python using string splitting, regular expressions, split() method, etc. Manipulating strings is a common task in programming, and extracting specific words from a string can be particularly useful in various scenarios. In this article, we will explore different methods to extract the Nth word from a given string using Python.
方法1:字串分割
這種方法涉及將字串分割為單字列表,並根據索引存取所需的單字。
Syntax
words = string.split()
Here, the split() method splits the string based on whitespace by default. The resulting words are stored in the words list.
演算法
Accept the input string and the value of N.
使用split()方法將字串拆分為單字清單。
Access the Nth word from the list using indexing.
Access the Nth word from the list using indexing.
Example
讓我們考慮以下字串:"The quick brown fox jumps over the lazy dog." 在下面的範例中,輸入字串使用split()方法分割成一個單字清單。由於N的值為3,函數傳回第三個單字,"brown"。
def get_nth_word_split(string, n): words = string.split() if 1 <= n <= len(words): return words[n-1] else: return "Invalid value of N." # Test the function input_string = "The quick brown fox jumps over the lazy dog." n = 3 print(get_nth_word_split(input_string, n))
輸出
brown
方法二:使用正規表示式
Another method to extract the Nth word involves using regular expressions. This approach provides flexibility in handling different word patterns and delimiters.
Syntax
import re words = re.findall(pattern, string)
在這裡,使用 re 模組中的 re.findall() 函數根據指定的模式從輸入字串中提取所有單字。模式是使用正規表示式定義的。提取到的單字儲存在 words 清單中。
演算法
導入 re 模組。
Accept the input string and the value of N.
定義一個正規表示式模式來符合單字。
Use the findall() function from the re module to extract all words from the string.
Access the Nth word from the list obtained in step 4 using indexing.
傳回第N個字。
Example
在下面的範例中,正規表示式模式'\w '用於匹配輸入字串中的所有單字。 findall()函數提取所有單字並將它們儲存在一個清單中。由於N的值為4,函數傳回第四個單字"jumps"。
import re def get_nth_word_regex(string, n): pattern = r'\w+' words = re.findall(pattern, string) if 1 <= n <= len(words): return words[n-1] else: return "Invalid value of N." # Test the function input_string = "The quick brown fox jumps over the lazy dog." n = 4 print(get_nth_word_regex(input_string, n))
輸出
fox
方法3:使用split()方法和自訂分隔符號
In some cases, the string may have a specific delimiter other than whitespace. In such scenarios, we can modify the first method by specifying a custom delimiter for splitting the string.
Syntax
words = string.split(delimiter)
在這裡,split()方法被用來根據自訂的分隔符號將輸入字串拆分為一個單字清單。分隔符號作為參數傳遞給split()方法。拆分後的單字儲存在words列表中。
演算法
Accept the input string, the delimiter, and the value of N.
使用split()方法和自訂分隔符號將字串分割成單字清單。
Access the Nth word from the list using indexing
傳回第N個字。
Example
在下面的範例中,字串使用自訂分隔符號(逗號“,”)被分割為單字。第二個單字“banana”被提取並返回,因為它對應於N的值為2。
def get_nth_word_custom_delimiter(string, delimiter, n): words = string.split(delimiter) if 1 <= n <= len(words): return words[n-1] else: return "Invalid value of N." # Test the function input_string = "apple,banana,orange,mango" delimiter = "," n = 2 print(get_nth_word_custom_delimiter(input_string, delimiter, n))
輸出
banana
Conclusion
在本文中,我們討論瞭如何借助拆分字串、使用正規表示式以及使用帶有自訂分隔符號的拆分方法來獲取給定字串中的第n個單字。每種方法都根據任務的要求提供了靈活性。透過理解和實施這些技術,您可以在Python程式中有效地提取字串中的特定單字。
以上是如何使用Python獲取給定字串中的第N個單字?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
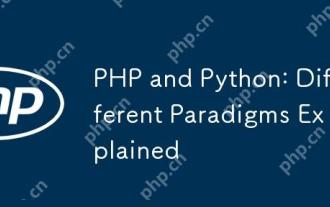
PHP主要是過程式編程,但也支持面向對象編程(OOP);Python支持多種範式,包括OOP、函數式和過程式編程。 PHP適合web開發,Python適用於多種應用,如數據分析和機器學習。
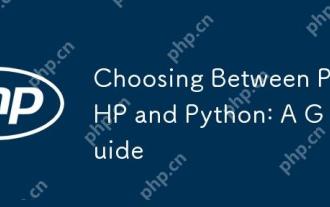
PHP適合網頁開發和快速原型開發,Python適用於數據科學和機器學習。 1.PHP用於動態網頁開發,語法簡單,適合快速開發。 2.Python語法簡潔,適用於多領域,庫生態系統強大。
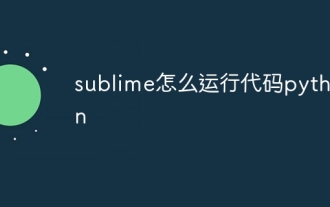
在 Sublime Text 中運行 Python 代碼,需先安裝 Python 插件,再創建 .py 文件並編寫代碼,最後按 Ctrl B 運行代碼,輸出會在控制台中顯示。
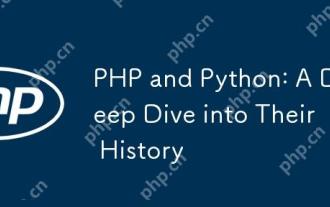
PHP起源於1994年,由RasmusLerdorf開發,最初用於跟踪網站訪問者,逐漸演變為服務器端腳本語言,廣泛應用於網頁開發。 Python由GuidovanRossum於1980年代末開發,1991年首次發布,強調代碼可讀性和簡潔性,適用於科學計算、數據分析等領域。
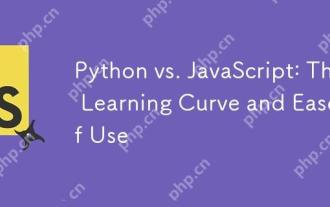
Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
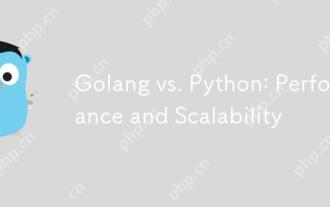
Golang在性能和可擴展性方面優於Python。 1)Golang的編譯型特性和高效並發模型使其在高並發場景下表現出色。 2)Python作為解釋型語言,執行速度較慢,但通過工具如Cython可優化性能。
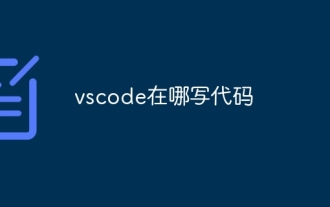
在 Visual Studio Code(VSCode)中編寫代碼簡單易行,只需安裝 VSCode、創建項目、選擇語言、創建文件、編寫代碼、保存並運行即可。 VSCode 的優點包括跨平台、免費開源、強大功能、擴展豐富,以及輕量快速。
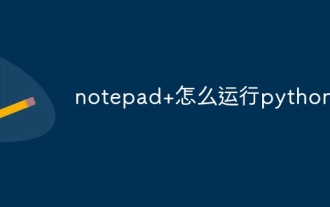
在 Notepad 中運行 Python 代碼需要安裝 Python 可執行文件和 NppExec 插件。安裝 Python 並為其添加 PATH 後,在 NppExec 插件中配置命令為“python”、參數為“{CURRENT_DIRECTORY}{FILE_NAME}”,即可在 Notepad 中通過快捷鍵“F6”運行 Python 代碼。
