탄력적인 마이크로서비스 설계: 클라우드 아키텍처에 대한 실용 가이드
최신 애플리케이션에는 확장성, 안정성 및 유지 관리 가능성이 필요합니다. 이 가이드에서는 운영 우수성을 유지하면서 실제 문제를 처리할 수 있는 마이크로서비스 아키텍처를 설계하고 구현하는 방법을 살펴보겠습니다.
기초: 서비스 디자인 원칙
우리 아키텍처를 안내하는 핵심 원칙부터 시작해 보겠습니다.
graph TD A[Service Design Principles] --> B[Single Responsibility] A --> C[Domain-Driven Design] A --> D[API First] A --> E[Event-Driven] A --> F[Infrastructure as Code]
탄력적인 서비스 구축
다음은 Go를 사용하여 잘 구성된 마이크로서비스의 예입니다.
package main import ( "context" "log" "net/http" "os" "os/signal" "syscall" "time" "github.com/prometheus/client_golang/prometheus" "go.opentelemetry.io/otel" ) // Service configuration type Config struct { Port string ShutdownTimeout time.Duration DatabaseURL string } // Service represents our microservice type Service struct { server *http.Server logger *log.Logger config Config metrics *Metrics } // Metrics for monitoring type Metrics struct { requestDuration *prometheus.HistogramVec requestCount *prometheus.CounterVec errorCount *prometheus.CounterVec } func NewService(cfg Config) *Service { metrics := initializeMetrics() logger := initializeLogger() return &Service{ config: cfg, logger: logger, metrics: metrics, } } func (s *Service) Start() error { // Initialize OpenTelemetry shutdown := initializeTracing() defer shutdown() // Setup HTTP server router := s.setupRoutes() s.server = &http.Server{ Addr: ":" + s.config.Port, Handler: router, } // Graceful shutdown go s.handleShutdown() s.logger.Printf("Starting server on port %s", s.config.Port) return s.server.ListenAndServe() }
회로 차단기 구현
연속적인 오류로부터 서비스를 보호하세요.
type CircuitBreaker struct { failureThreshold uint32 resetTimeout time.Duration state uint32 failures uint32 lastFailure time.Time } func NewCircuitBreaker(threshold uint32, timeout time.Duration) *CircuitBreaker { return &CircuitBreaker{ failureThreshold: threshold, resetTimeout: timeout, } } func (cb *CircuitBreaker) Execute(fn func() error) error { if !cb.canExecute() { return errors.New("circuit breaker is open") } err := fn() if err != nil { cb.recordFailure() return err } cb.reset() return nil }
이벤트 중심 커뮤니케이션
신뢰할 수 있는 이벤트 스트리밍을 위해 Apache Kafka 사용:
type EventProcessor struct { consumer *kafka.Consumer producer *kafka.Producer logger *log.Logger } func (ep *EventProcessor) ProcessEvents(ctx context.Context) error { for { select { case <-ctx.Done(): return ctx.Err() default: msg, err := ep.consumer.ReadMessage(ctx) if err != nil { ep.logger.Printf("Error reading message: %v", err) continue } if err := ep.handleEvent(ctx, msg); err != nil { ep.logger.Printf("Error processing message: %v", err) // Handle dead letter queue ep.moveToDeadLetter(msg) } } } }
코드로서의 인프라
인프라 관리를 위해 Terraform 사용:
# Define the microservice infrastructure module "microservice" { source = "./modules/microservice" name = "user-service" container_port = 8080 replicas = 3 environment = { KAFKA_BROKERS = var.kafka_brokers DATABASE_URL = var.database_url LOG_LEVEL = "info" } # Configure auto-scaling autoscaling = { min_replicas = 2 max_replicas = 10 metrics = [ { type = "Resource" resource = { name = "cpu" target_average_utilization = 70 } } ] } } # Set up monitoring module "monitoring" { source = "./modules/monitoring" service_name = module.microservice.name alert_email = var.alert_email dashboard = { refresh_interval = "30s" time_range = "6h" } }
OpenAPI를 사용한 API 설계
서비스 API 계약 정의:
openapi: 3.0.3 info: title: User Service API version: 1.0.0 description: User management microservice API paths: /users: post: summary: Create a new user operationId: createUser requestBody: required: true content: application/json: schema: $ref: '#/components/schemas/CreateUserRequest' responses: '201': description: User created successfully content: application/json: schema: $ref: '#/components/schemas/User' '400': $ref: '#/components/responses/BadRequest' '500': $ref: '#/components/responses/InternalError' components: schemas: User: type: object properties: id: type: string format: uuid email: type: string format: email created_at: type: string format: date-time required: - id - email - created_at
관찰 가능성 구현
종합 모니터링 설정:
# Prometheus configuration scrape_configs: - job_name: 'microservices' kubernetes_sd_configs: - role: pod relabel_configs: - source_labels: [__meta_kubernetes_pod_annotation_prometheus_io_scrape] action: keep regex: true # Grafana dashboard { "dashboard": { "panels": [ { "title": "Request Rate", "type": "graph", "datasource": "Prometheus", "targets": [ { "expr": "rate(http_requests_total{service=\"user-service\"}[5m])", "legendFormat": "{{method}} {{path}}" } ] }, { "title": "Error Rate", "type": "graph", "datasource": "Prometheus", "targets": [ { "expr": "rate(http_errors_total{service=\"user-service\"}[5m])", "legendFormat": "{{status_code}}" } ] } ] } }
배포 전략
다운타임 없는 배포 구현:
apiVersion: apps/v1 kind: Deployment metadata: name: user-service spec: replicas: 3 strategy: type: RollingUpdate rollingUpdate: maxSurge: 1 maxUnavailable: 0 template: spec: containers: - name: user-service image: user-service:1.0.0 ports: - containerPort: 8080 readinessProbe: httpGet: path: /health port: 8080 initialDelaySeconds: 5 periodSeconds: 10 livenessProbe: httpGet: path: /health port: 8080 initialDelaySeconds: 15 periodSeconds: 20
생산 모범 사례
- 적절한 상태 점검 및 준비 상태 조사 구현
- 상관 ID와 함께 구조화된 로깅 사용
- 지수 백오프로 적절한 재시도 정책 구현
- 외부 종속성을 위해 회로 차단기 사용
- 적절한 속도 제한 구현
- 주요 측정항목 모니터링 및 알림
- 적절한 비밀 관리 사용
- 적절한 백업 및 재해 복구 구현
결론
복원력이 뛰어난 마이크로서비스를 구축하려면 여러 요소를 신중하게 고려해야 합니다. 핵심은 다음과 같습니다.
- 실패를 고려한 디자인
- 적절한 관찰 가능성 구현
- 코드형 인프라 사용
- 적절한 테스트 전략 구현
- 적절한 배포 전략 사용
- 효과적인 모니터링 및 알림
마이크로서비스를 구축하면서 어떤 어려움을 겪었나요? 아래 댓글로 여러분의 경험을 공유해 주세요!
위 내용은 탄력적인 마이크로서비스 설계: 클라우드 아키텍처에 대한 실용 가이드의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
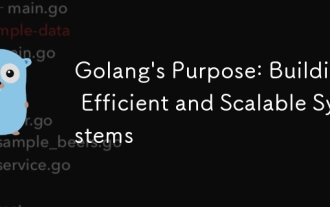
Go Language는 효율적이고 확장 가능한 시스템을 구축하는 데 잘 작동합니다. 장점은 다음과 같습니다. 1. 고성능 : 기계 코드로 컴파일, 빠른 달리기 속도; 2. 동시 프로그래밍 : 고어 라틴 및 채널을 통한 멀티 태스킹 단순화; 3. 단순성 : 간결한 구문, 학습 및 유지 보수 비용 절감; 4. 크로스 플랫폼 : 크로스 플랫폼 컴파일, 쉬운 배포를 지원합니다.
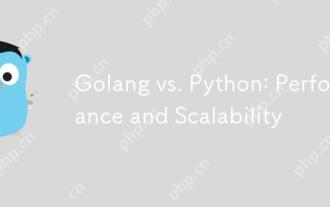
Golang은 성능과 확장 성 측면에서 Python보다 낫습니다. 1) Golang의 컴파일 유형 특성과 효율적인 동시성 모델은 높은 동시성 시나리오에서 잘 수행합니다. 2) 해석 된 언어로서 파이썬은 천천히 실행되지만 Cython과 같은 도구를 통해 성능을 최적화 할 수 있습니다.
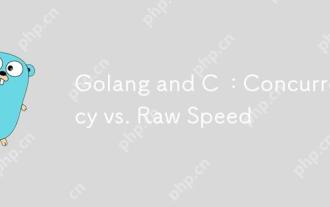
Golang은 동시성에서 C보다 낫고 C는 원시 속도에서 Golang보다 낫습니다. 1) Golang은 Goroutine 및 Channel을 통해 효율적인 동시성을 달성하며, 이는 많은 동시 작업을 처리하는 데 적합합니다. 2) C 컴파일러 최적화 및 표준 라이브러리를 통해 하드웨어에 가까운 고성능을 제공하며 극도의 최적화가 필요한 애플리케이션에 적합합니다.
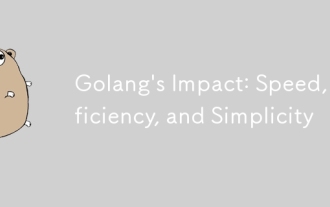
goimpactsdevelopmentpositively throughlyspeed, 효율성 및 단순성.
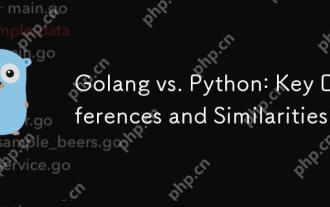
Golang과 Python은 각각 고유 한 장점이 있습니다. Golang은 고성능 및 동시 프로그래밍에 적합하지만 Python은 데이터 과학 및 웹 개발에 적합합니다. Golang은 동시성 모델과 효율적인 성능으로 유명하며 Python은 간결한 구문 및 풍부한 라이브러리 생태계로 유명합니다.
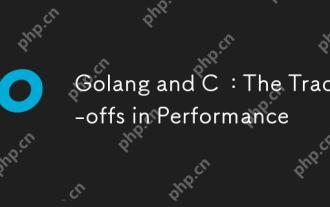
Golang과 C의 성능 차이는 주로 메모리 관리, 컴파일 최적화 및 런타임 효율에 반영됩니다. 1) Golang의 쓰레기 수집 메커니즘은 편리하지만 성능에 영향을 줄 수 있습니다. 2) C의 수동 메모리 관리 및 컴파일러 최적화는 재귀 컴퓨팅에서 더 효율적입니다.
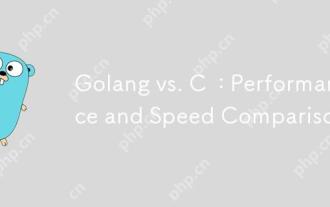
Golang은 빠른 개발 및 동시 시나리오에 적합하며 C는 극도의 성능 및 저수준 제어가 필요한 시나리오에 적합합니다. 1) Golang은 쓰레기 수집 및 동시성 메커니즘을 통해 성능을 향상시키고, 고전성 웹 서비스 개발에 적합합니다. 2) C는 수동 메모리 관리 및 컴파일러 최적화를 통해 궁극적 인 성능을 달성하며 임베디드 시스템 개발에 적합합니다.
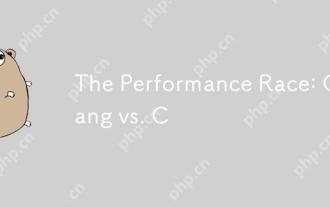
Golang과 C는 각각 공연 경쟁에서 고유 한 장점을 가지고 있습니다. 1) Golang은 높은 동시성과 빠른 발전에 적합하며 2) C는 더 높은 성능과 세밀한 제어를 제공합니다. 선택은 프로젝트 요구 사항 및 팀 기술 스택을 기반으로해야합니다.
