Go をマスターする: 最新の Golang 開発の実践ガイド
Go は、最新のバックエンド開発、クラウド サービス、DevOps ツールの原動力となっています。言語の長所を活用した慣用的な Go コードの書き方を見てみましょう。
Go 環境のセットアップ
まず、最新の Go プロジェクト構造をセットアップしましょう:
# Initialize a new module go mod init myproject # Project structure myproject/ ├── cmd/ │ └── api/ │ └── main.go ├── internal/ │ ├── handlers/ │ ├── models/ │ └── services/ ├── pkg/ │ └── utils/ ├── go.mod └── go.sum
クリーンな Go コードの作成
これは、適切に構造化された Go プログラムの例です。
package main import ( "context" "log" "net/http" "os" "os/signal" "syscall" "time" ) // Server configuration type Config struct { Port string ReadTimeout time.Duration WriteTimeout time.Duration ShutdownTimeout time.Duration } // Application represents our web server type Application struct { config Config logger *log.Logger router *http.ServeMux } // NewApplication creates a new application instance func NewApplication(cfg Config) *Application { logger := log.New(os.Stdout, "[API] ", log.LstdFlags) return &Application{ config: cfg, logger: logger, router: http.NewServeMux(), } } // setupRoutes configures all application routes func (app *Application) setupRoutes() { app.router.HandleFunc("/health", app.healthCheckHandler) app.router.HandleFunc("/api/v1/users", app.handleUsers) } // Run starts the server and handles graceful shutdown func (app *Application) Run() error { // Setup routes app.setupRoutes() // Create server srv := &http.Server{ Addr: ":" + app.config.Port, Handler: app.router, ReadTimeout: app.config.ReadTimeout, WriteTimeout: app.config.WriteTimeout, } // Channel to listen for errors coming from the listener. serverErrors := make(chan error, 1) // Start the server go func() { app.logger.Printf("Starting server on port %s", app.config.Port) serverErrors <- srv.ListenAndServe() }() // Listen for OS signals shutdown := make(chan os.Signal, 1) signal.Notify(shutdown, os.Interrupt, syscall.SIGTERM) // Block until we receive a signal or an error select { case err := <-serverErrors: return fmt.Errorf("server error: %w", err) case <-shutdown: app.logger.Println("Starting shutdown...") // Create context for shutdown ctx, cancel := context.WithTimeout( context.Background(), app.config.ShutdownTimeout, ) defer cancel() // Gracefully shutdown the server err := srv.Shutdown(ctx) if err != nil { return fmt.Errorf("graceful shutdown failed: %w", err) } } return nil }
インターフェイスの操作とエラー処理
Go のインターフェース システムとエラー処理は重要な機能です:
// UserService defines the interface for user operations type UserService interface { GetUser(ctx context.Context, id string) (*User, error) CreateUser(ctx context.Context, user *User) error UpdateUser(ctx context.Context, user *User) error DeleteUser(ctx context.Context, id string) error } // Custom error types type NotFoundError struct { Resource string ID string } func (e *NotFoundError) Error() string { return fmt.Sprintf("%s with ID %s not found", e.Resource, e.ID) } // Implementation type userService struct { db *sql.DB logger *log.Logger } func (s *userService) GetUser(ctx context.Context, id string) (*User, error) { user := &User{} err := s.db.QueryRowContext( ctx, "SELECT id, name, email FROM users WHERE id = ", id, ).Scan(&user.ID, &user.Name, &user.Email) if err == sql.ErrNoRows { return nil, &NotFoundError{Resource: "user", ID: id} } if err != nil { return nil, fmt.Errorf("querying user: %w", err) } return user, nil }
同時実行パターン
Go のゴルーチンとチャネルにより、同時プログラミングが簡単になります:
// Worker pool pattern func processItems(items []string, numWorkers int) error { jobs := make(chan string, len(items)) results := make(chan error, len(items)) // Start workers for w := 0; w < numWorkers; w++ { go worker(w, jobs, results) } // Send jobs to workers for _, item := range items { jobs <- item } close(jobs) // Collect results for range items { if err := <-results; err != nil { return err } } return nil } func worker(id int, jobs <-chan string, results chan<- error) { for item := range jobs { results <- processItem(item) } } // Rate limiting func rateLimiter[T any](input <-chan T, limit time.Duration) <-chan T { output := make(chan T) ticker := time.NewTicker(limit) go func() { defer close(output) defer ticker.Stop() for item := range input { <-ticker.C output <- item } }() return output }
テストとベンチマーク
Go には優れたテストサポートが組み込まれています:
// user_service_test.go package service import ( "context" "testing" "time" ) func TestUserService(t *testing.T) { // Table-driven tests tests := []struct { name string userID string want *User wantErr bool }{ { name: "valid user", userID: "123", want: &User{ ID: "123", Name: "Test User", }, wantErr: false, }, { name: "invalid user", userID: "999", want: nil, wantErr: true, }, } for _, tt := range tests { t.Run(tt.name, func(t *testing.T) { svc := NewUserService(testDB) got, err := svc.GetUser(context.Background(), tt.userID) if (err != nil) != tt.wantErr { t.Errorf("GetUser() error = %v, wantErr %v", err, tt.wantErr) return } if !reflect.DeepEqual(got, tt.want) { t.Errorf("GetUser() = %v, want %v", got, tt.want) } }) } } // Benchmarking example func BenchmarkUserService_GetUser(b *testing.B) { svc := NewUserService(testDB) ctx := context.Background() b.ResetTimer() for i := 0; i < b.N; i++ { _, _ = svc.GetUser(ctx, "123") } }
パフォーマンスの最適化
Go を使用すると、コードのプロファイリングと最適化が簡単になります:
// Use sync.Pool for frequently allocated objects var bufferPool = sync.Pool{ New: func() interface{} { return new(bytes.Buffer) }, } func processRequest(data []byte) string { buf := bufferPool.Get().(*bytes.Buffer) defer bufferPool.Put(buf) buf.Reset() buf.Write(data) // Process data... return buf.String() } // Efficiently handle JSON type User struct { ID string `json:"id"` Name string `json:"name"` Email string `json:"email"` CreatedAt time.Time `json:"created_at"` } func (u *User) MarshalJSON() ([]byte, error) { type Alias User return json.Marshal(&struct { *Alias CreatedAt string `json:"created_at"` }{ Alias: (*Alias)(u), CreatedAt: u.CreatedAt.Format(time.RFC3339), }) }
本番環境のベストプラクティス
- 適切なコンテキスト管理を使用する
- 正常なシャットダウンを実装する
- 適切なエラー処理を使用する
- 適切なロギングを実装する
- 依存性注入を使用する
- 包括的なテストを作成する
- パフォーマンスのプロファイリングと最適化
- 適切なプロジェクト構造を使用する
結論
Go のシンプルさと強力な機能により、Go は最新の開発に最適です。重要なポイント:
- 慣用的な Go コード スタイルに従います
- 抽象化のためにインターフェイスを使用する
- Go の同時実行機能を活用する
- 包括的なテストを作成する
- パフォーマンスに重点を置く
- 適切なプロジェクト構造を使用する
Go 開発のどの側面に最も興味がありますか?以下のコメント欄であなたの経験を共有してください!
以上がGo をマスターする: 最新の Golang 開発の実践ガイドの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










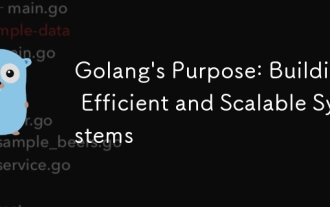
GO言語は、効率的でスケーラブルなシステムの構築においてうまく機能します。その利点には次のものがあります。1。高性能:マシンコードにコンパイルされ、速度速度が速い。 2。同時プログラミング:ゴルチンとチャネルを介してマルチタスクを簡素化します。 3。シンプルさ:簡潔な構文、学習コストとメンテナンスコストの削減。 4。クロスプラットフォーム:クロスプラットフォームのコンパイル、簡単な展開をサポートします。
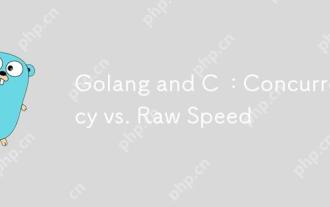
Golangは並行性がCよりも優れていますが、Cは生の速度ではGolangよりも優れています。 1)Golangは、GoroutineとChannelを通じて効率的な並行性を達成します。これは、多数の同時タスクの処理に適しています。 2)Cコンパイラの最適化と標準ライブラリを介して、極端な最適化を必要とするアプリケーションに適したハードウェアに近い高性能を提供します。
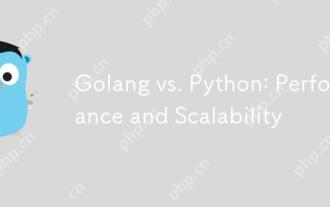
Golangは、パフォーマンスとスケーラビリティの点でPythonよりも優れています。 1)Golangのコンピレーションタイプの特性と効率的な並行性モデルにより、高い並行性シナリオでうまく機能します。 2)Pythonは解釈された言語として、ゆっくりと実行されますが、Cythonなどのツールを介してパフォーマンスを最適化できます。
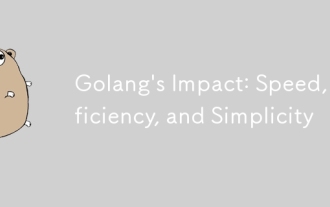
speed、効率、およびシンプル性をspeedsped.1)speed:gocompilesquilesquicklyandrunseffictient、理想的なlargeprojects.2)効率:等系dribribraryreducesexexternaldedenciess、開発効果を高める3)シンプルさ:
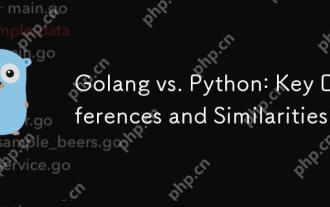
GolangとPythonにはそれぞれ独自の利点があります。Golangは高性能と同時プログラミングに適していますが、PythonはデータサイエンスとWeb開発に適しています。 Golangは同時性モデルと効率的なパフォーマンスで知られていますが、Pythonは簡潔な構文とリッチライブラリエコシステムで知られています。
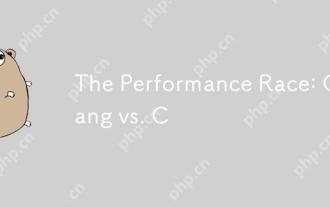
GolangとCにはそれぞれパフォーマンス競争において独自の利点があります。1)Golangは、高い並行性と迅速な発展に適しており、2)Cはより高いパフォーマンスと微細な制御を提供します。選択は、プロジェクトの要件とチームテクノロジースタックに基づいている必要があります。
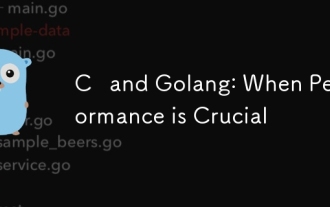
Cは、ハードウェアリソースと高性能の最適化が必要なシナリオにより適していますが、Golangは迅速な開発と高い並行性処理が必要なシナリオにより適しています。 1.Cの利点は、ハードウェア特性と高い最適化機能に近いものにあります。これは、ゲーム開発などの高性能ニーズに適しています。 2.Golangの利点は、その簡潔な構文と自然な並行性サポートにあり、これは高い並行性サービス開発に適しています。
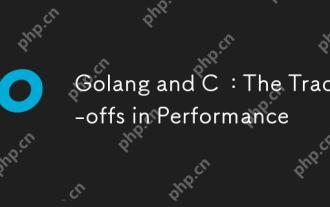
GolangとCのパフォーマンスの違いは、主にメモリ管理、コンピレーションの最適化、ランタイム効率に反映されています。 1)Golangのゴミ収集メカニズムは便利ですが、パフォーマンスに影響を与える可能性があります。
